Addition of two matrices in Python
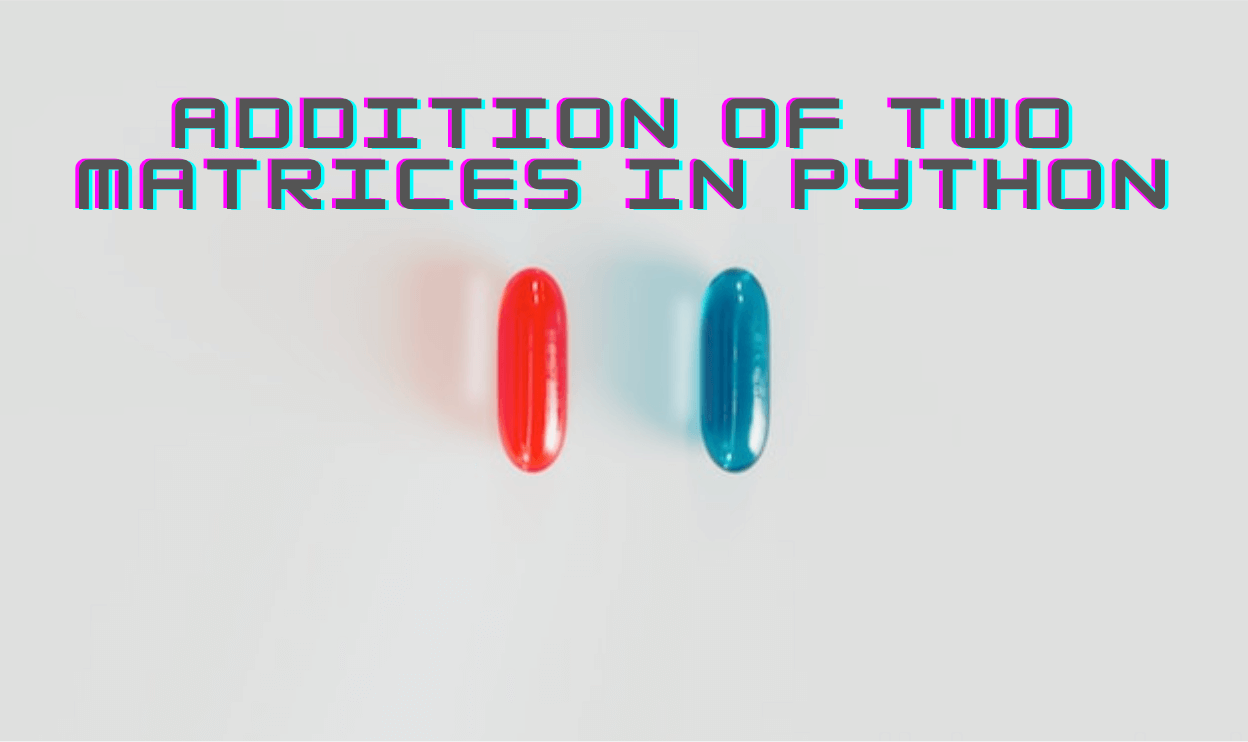
Matrices are a mathematical concept used in various fields in the real world such as physics, engineering, etc. In Python, We can able to perform an arithmetic operation on top of matrices. In this article, we will learn about the addition of two matrices in Python.
Adding two matrices in Python is a simple task that can be accomplished using the np.add() function from the NumPy library. It takes two matrices as inputs and returns a new matrix that represents the sum of the original matrices.
There are multiple other ways to achieve the addition of two matrices which have been discussed in this article as well
What are Matrices?
A matrix is a two-dimensional array of numbers or values arranged in rows and columns. In Python, the matrix is represented as a list of lists, where each inner list represents a row of the matrix. Consider the below matrix as an example: which is a 3×3 matrix (3 rows and 3 columns)
1 2 3 4 5 6 7 8 9
As mentioned, in Python the matrics is represented as a list of lists as shown below
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
Adding Two Matrices in Python can be done by following the methods
- Nested Loop
- Using NumPy
- Using List Comprehension
- Using the Zip Function
- Using the map Function
Nested Loop
In this method, We will be using a nested loop to add two matrices
Example:
# Define the matrices matrix1 = [[1, 2, 3], [4, 5, 6], [7, 8, 9]] matrix2 = [[10, 11, 12], [13, 14, 15], [16, 17, 18]] # Create an empty matrix to store the result result = [[0, 0, 0], [0, 0, 0], [0, 0, 0]] # Iterate over each element of the matrices and add them together for i in range(len(matrix1)): for j in range(len(matrix1[0])): result[i][j] = matrix1[i][j] + matrix2[i][j] # Print the result for row in result: print(row)
In the above example, we have defined two matrices matrix1
and matrix2
with the same dimensions (3×3). Then we created an empty matrix result
with the same dimensions to store the result of the addition.
Once done, we can now iterate over each element of the matrices using nested loops and add the corresponding elements together and store it in the result
matrix. At last, we can able to print the result
matrix to see the sum of values.
Output
[11, 13, 15] [17, 19, 21] [23, 25, 27] Process finished with exit code 0
Using NumPy
In this method, We are going to add two numbers using the Numpy module and it is considered an efficient method for large matrices as it provides optimized operations.
Steps to install NumPy: In this article, I have used pycharm IDE to execute the example code, To install Numpy module in pycharm, follow the below steps
Pycharm -> preferences -> project -> Python interpreter -> Add module -> search "numpy" -> install

If you are running the example in the terminal, we can use pip to install the NumPy module
pip install numpy
“+” Operator
Example:
import numpy as np # define the matrices as NumPy arrays matrix1 = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]]) matrix2 = np.array([[10, 11, 12], [13, 14, 15], [16, 17, 18]]) # add the matrices using the '+' operator result = matrix1 + matrix2 # print the result print(result)
In the above example, we are importing the NumPy library and defining the two matrices using the np.array()
function. Once it is defined, We can add the two matrices using the ‘+’ operator and store the result in the result
variable and can be printed to see the final output
Output
[[11 13 15] [17 19 21] [23 25 27]] Process finished with exit code 0
numpy.add()
We can also add two matrices in Python by using the numpy.add()
function
import numpy as np # define the matrices as NumPy arrays matrix1 = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]]) matrix2 = np.array([[10, 11, 12], [13, 14, 15], [16, 17, 18]]) # add the matrices using the numpy add function result = np.add(matrix1, matrix2) # print the result print(result)
It is similar to the (+) operator shown in the above example, Instead of + we are using numpy.add() the function to add the two matrices together and store the result in the result
variable. At last, we print the result
matrix.
Output:
[[11 13 15] [17 19 21] [23 25 27]] Process finished with exit code 0
Using List Comprehension
In this method, We are using list comprehension to add two matrices in Python.
Example:
# define the matrices matrix1 = [[1, 2, 3], [4, 5, 6], [7, 8, 9]] matrix2 = [[10, 11, 12], [13, 14, 15], [16, 17, 18]] # add the matrices using list comprehension result = [[matrix1[i][j] + matrix2[i][j] for j in range(len(matrix1[0]))] for i in range(len(matrix1))] # print the result for row in result: print(row)
In the above example, we are using nested list comprehension to add the two matrices. Initially, we define the two matrices as a variable, then use list comprehension to iterate over each element of the matrices and add them together, and stored them in the variable result, We then print the result
matrix to see the final result.
Output:
[11, 13, 15] [17, 19, 21] [23, 25, 27] Process finished with exit code 0
Using the Zip Function
In this method, We are using zip()
function to add two matrices in Python.
Example:
# define the matrices matrix1 = [[1, 2, 3], [4, 5, 6], [7, 8, 9]] matrix2 = [[10, 11, 12], [13, 14, 15], [16, 17, 18]] # add the matrices using the zip function result = [[x + y for x, y in zip(row1, row2)] for row1, row2 in zip(matrix1, matrix2)] # print the result for row in result: print(row)
In the above example, we are using the zip()
function to iterate over each row of the matrices simultaneously and add the corresponding elements together using list comprehension. We then store the result in the result
matrix and print it.
Output:
[11, 13, 15] [17, 19, 21] [23, 25, 27] Process finished with exit code 0
Using the map Function
In this method, We are going to use the map()
function to add two matrices in Python.
Example:
# define the matrices matrix1 = [[1, 2, 3], [4, 5, 6], [7, 8, 9]] matrix2 = [[10, 11, 12], [13, 14, 15], [16, 17, 18]] # add the matrices using the map function result = list(map(lambda x, y: list(map(sum, zip(x, y))), matrix1, matrix2)) # print the result for row in result: print(row)
In the above example, we use the map()
function along with lambda
functions to add the two matrices. Initially, We use map()
to iterate over each row, then use zip()
to combine the corresponding elements of each row, use sum()
to add them together. Once down, We can convert the resulting map object to a list and store it in the result
matrix which can be printed
Output:
[11, 13, 15] [17, 19, 21] [23, 25, 27] Process finished with exit code 0
Conclusion
In conclusion, adding two matrices in Python is the most used operation that comes up in multiple use cases. With the above-discussed methods, you should now be able to have an understanding of how to perform this operation and which method to use in which use case.
Good Luck with your Learning !!
Related Topics:
What datatype is represented by int in python 3
How to Use Python Print to stderr
Coding Spell | Python Journey | About Us
- How to Fix – TypeError: only size-1 arrays can be converted to Python scalars - 16 October 2023
- How to Implement d’wave qbsolv in Python - 16 October 2023
- Resolve Javascript error: ipython is not defined - 15 October 2023