Write a Program to Reverse a Number in Python
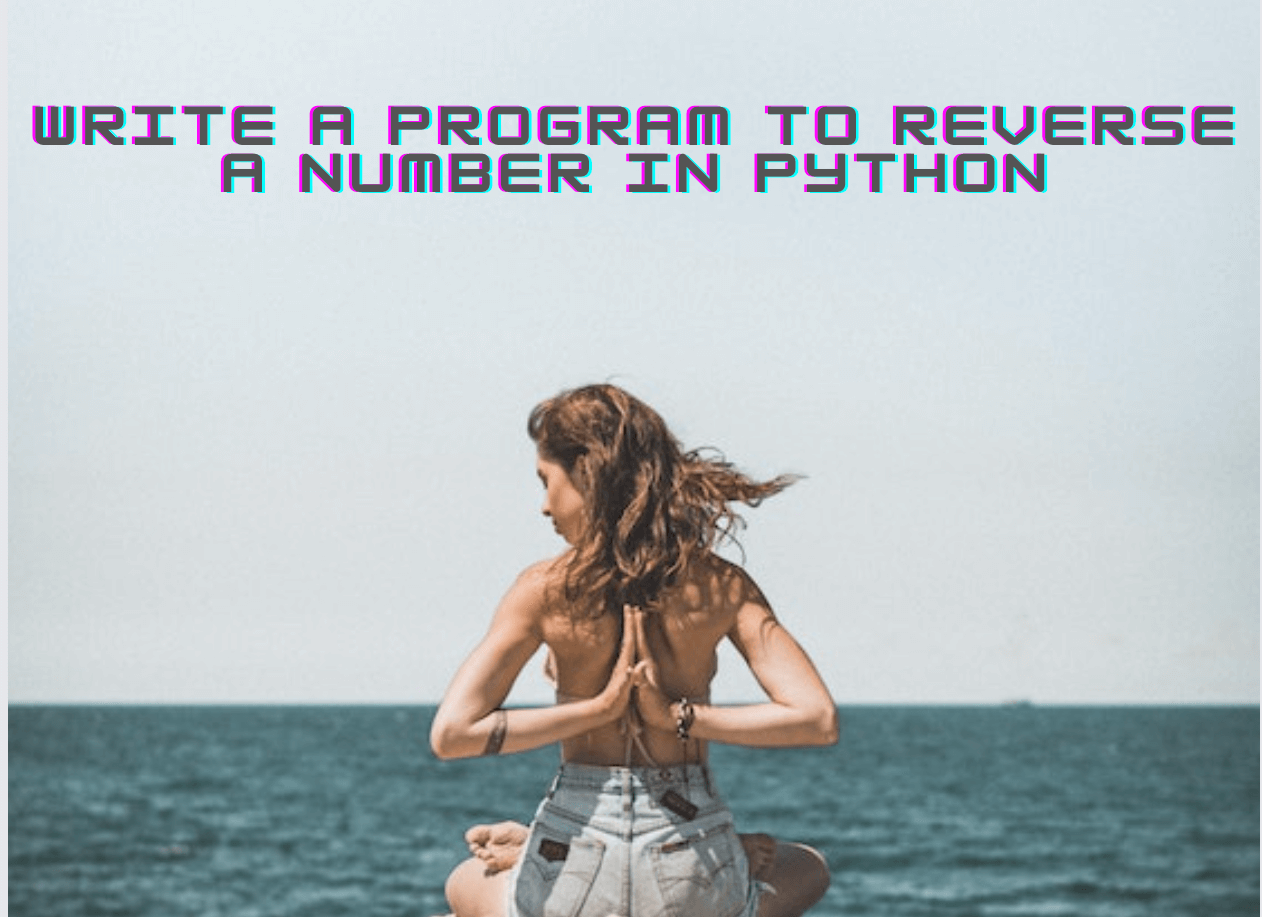
Ever wanted to flip numbers backward just for fun? Like turning 123 into 321? Well, writing a Python program to reverse a number is not only a playful exercise but also a good way to get comfortable with the number and string manipulation in code! So, let’s dive into how we can Reverse numbers around with a few lines of Python!
Reverse a number is a common programming task that involves reversing the order of the digits in a given number. We are going to learn different techniques in this article including reversing the number using a while loop, Slicing, and the reversed() method to create a reversed iterable object
12345 => 54321
Introduction
Python is a popular programming language that offers various techniques to perform this task efficiently. In this article, we will explore different ways to reverse a number in Python using code examples. We will walk through step-by-step explanations and provide code snippets to demonstrate each method. Whether you are new to programming or an experienced developer, this article will provide you with the knowledge you need to reverse a number in Python.
There are several ways to reverse a number in Python. Here are a few examples:
Using While Loop
number = 12345
reverse_number = 0
while number > 0:
digit = number % 10
reverse_number = (reverse_number * 10) + digit
number //= 10
print(reverse_number)
Output
54321
The detailed description of the code is as follows:
- We first initialize the variables number to 12345 and rev_number to 0.
- In the first iteration of the while loop, the modulus operator % is used to get the last digit of number, which is 5. The variable digit is set to 5.
- The reversed number reverse_number is updated by multiplying the current value of reverse_number by 10 and adding the current digit. So, reverse_number becomes 5.
- The last digit is removed from number by using integer division //, which sets number to 1234.
- The while loop continues to run until number becomes 0. In the second iteration, digit becomes 4 and reverse_number becomes 54.
- In the third iteration, digit becomes 3 and reverse_number becomes 543.
- In the fourth iteration, digit becomes 2 and reverse_number becomes 5432.
- In the fifth and final iteration, digit becomes 1 and reverse_number becomes 54321.
- The while loop condition number > 0 is no longer true, so the loop ends.
- The reversed number reverse_number is printed, which is 54321.
- So, the output of the program is the reversed number of the original input number 12345, which is 54321.
Let’s dry run the above code to understand step-by-step calculation using the formulas:
Iteration | num | rem = num % 10 | reverse = reverse * 10 + rem | num //= 10 |
---|---|---|---|---|
1 | 12345 | 12345 % 10 = 5 | 0 * 10 + 5 = 5 | 12345 // 10 = 1234 |
2 | 1234 | 1234 % 10 = 4 | 5 * 10 + 4 = 54 | 1234 // 10 =123 |
3 | 123 | 123 % 10 = 3 | 54 * 10 + 3 = 543 | 123 // 10 = 12 |
4 | 12 | 12% 10 = 2 | 543 * 10 + 2 = 5432 | 12 // 10 = 1 |
5 | 1 | 1 % 10 = 1 | 5432 * 10 + 1 = 54321 (final ans) | 1 // 10 = 0 |
Using Slicing
num = 12345
rev_num = str(num)[::-1]
print(int(rev_num))
Output
54321
This code is an example of how to reverse a number in Python using string slicing. Here’s how it works:
- We first define the variable num and assign it the value 12345.
- Next, we convert the integer num into a string using the str() function.
- We use string slicing to reverse the string. The syntax [start:stop:step] is used for string slicing, where start is the starting index (which defaults to 0 if not specified), stop is the stopping index (which defaults to the end of the string if not specified), and step is the step value (which defaults to 1 if not specified). In this case, we leave start and stop blank, and set step to -1, which tells Python to start from the end of the string and move backward. So, [::-1] reverses the string.
- We assign the reversed string to the variable rev_num.
- Finally, we convert the reversed string back to an integer using the int() function and print the result.
When we run this code, we get the output 54321, which is the reversed number of the original input number 12345.
Using reversed() Method:
num = input("Enter a number to be reversed: ")
rev_num = int("".join(reversed(num)))
print("The reversed number is:", rev_num)
Output
Enter a number to be reversed: 54321
The reversed number is: 12345
A step-by-step explanation of the above code:
- The input() function is used to prompt the user to enter a number.
- The user’s input is stored in the variable num as a string.
- The reversed() function is applied to the string to create a reversed iterable object containing the characters of the string in reverse order.
- The join() method is called on an empty string “” to concatenate the characters of the iterable object into a single string.
- The resulting string, which contains the digits of the input number in reverse order, is converted back to an integer using the int() function.
- The reversed number is stored in a variable named rev_num.
- The print() function is used to display the reversed number to the console.
In summary, this code reverses the digits of a given number and prints the result to the console. When executed with the initial value of num set to 12345, it outputs the reversed number 54321.
Using Recursion
def reverse(number):
if number == 0:
return 0
return (number % 10 * (10 ** len(str(number))) // 10) + reverse(number // 10)
number = 12345
reverse_number = reverse(number)
print(reverse_number)
Output
54321
Here’s a step-by-step explanation of the code:
- A function named reverse is defined that takes a single argument number.
- The function first checks if the value of number is equal to 0. If so, it immediately returns 0.
- If number is not 0, the function computes the value of the last digit of number by taking the remainder of number divided by 10, using the modulus operator %.
- It then computes the length of number by converting it to a string using the str() function and calling the len() function on the resulting string.
- The function then computes the reversed number recursively by calling itself with the remaining digits of number (excluding the last digit) as the argument and adding the last digit to the result multiplied by 10 to the power of the length of number minus 1 (to account for the position of the last digit).
- Once the recursion has reached the base case (i.e., number equals 0), the function returns the final reversed number.
- The variable number is assigned the value 12345.
- The function reverse is called with number as its argument, and the resulting reversed number is stored in the variable reverse_number.
- The print() function is used to display the reversed number to the console.
Overall, this code reverses the digits of a given number using recursion and prints the result to the console. When executed with the initial value of the number set to 12345, it outputs the reversed number 54321.
Conclusion
In conclusion, reversing a number in Python can be achieved using different techniques. The methods discussed in this article include reversing the number using a while loop, Slicing, the reversed() method to create a reversed iterable object, and implementing a recursive function to reverse the digits of the number. By understanding these methods, programmers can choose the most suitable approach according to their specific needs.
Good Luck with your Learning !!
Related Topics:
Addition of two matrices in Python
How to Draw a Triangle in Python Turtle
What datatype is represented by int in python 3
Coding Spell | Python Journey | About Us
- How to Fix – TypeError: only size-1 arrays can be converted to Python scalars - 16 October 2023
- How to Implement d’wave qbsolv in Python - 16 October 2023
- Resolve Javascript error: ipython is not defined - 15 October 2023