What does pass do in Python?
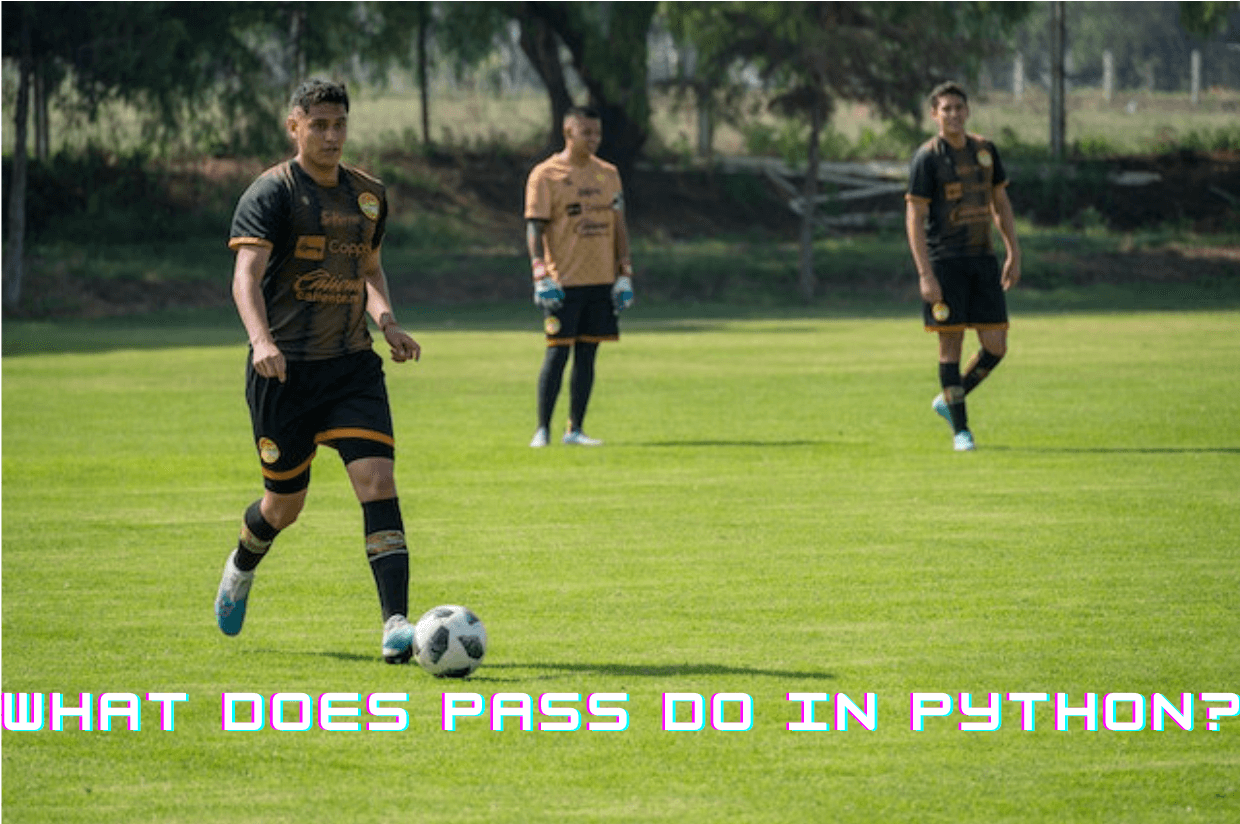
As a programming language, Python provides a wide range of statements and expressions to control the flow of execution in your code, Specifically, We going to see, What does pass do in Python?
pass is a null statement that does nothing when executed. It serves as a placeholder in situations where a statement is required for syntactical correctness, but no action is desired. If the interpreter read the line of code where it encounters a pass statement, it will skip that line and will jump to the next line.
Consider a scenario where you want to define a function called my_func(), but you haven’t decided on its functionality yet. Let’s see how python will respond in this case:
def my_func():
# some code here
Output:

We can observe that the above code generated an error because in Python functions, condition statements, loops, and classes cannot be defined without code. And if we try to do this, an error will be thrown as shown in the above case.
In situations where you need to have an empty code block but want to avoid errors, you can put the pass statement as a placeholder and come back later to fill in the code.
Let’s re-write the above my_func() function:
def my_func():
# some code here
pass
In this example, the pass statement is executed when the function is defined, but it does nothing. The function my_func() is defined, but it has no code to be executed when it is called.
Running the above example will not provide you with any output or ERROR, it simply passes the function without doing anything
Use Cases of Pass:
Here are some common use cases for the pass statement in Python:
Use as a placeholder in incomplete code: When you’re working on a new feature or code snippet and don’t have the complete implementation ready yet, you can use the pass statement as a placeholder.
Here’s an example of using the pass statement as a placeholder in incomplete code:
def new_feature():
# some code here
pass
# more code here
# implementation for new_feature is not yet complete, so a pass statement is used as a placeholder
In Functions or Classes with no implementation: If you want to define a function or class that doesn’t have any implementation, you can use the pass statement within the definition.
- In a function definition:
def my_function():
pass
# my_function definition is not complete, so a pass statement is used as a placeholder
- In a class definition:
class MyClass:
pass
# MyClass definition is not complete, so a pass statement is used as a placeholder
In conditional statements: When you want to do something when a certain condition is met, but you haven’t decided yet what that should be. The pass statement can be used.
num = -7
if num < 0:
pass
In this example, the pass statement is executed when num is less than 0, but it does nothing.
In loops: When you want to implement a loop to perform a certain action but haven’t decided yet what that action should be, you can put the pass statement as a placeholder.
- In a loop as a placeholder for future implementation:
for item in items:
pass
# implementation for the loop is not yet complete, so a pass statement is used as a placeholder
- In a loop to skip an iteration:
for item in items:
if item == 'laptop':
pass
else:
# perform some action
# skip an iteration if the current item is equal to 'laptop'
These are just a few examples of the use of the pass statement in Python. The statement can be used in a variety of other situations as well, where you need to include a statement in your code but don’t want it to do anything just yet.
Note: The difference between a comment and a pass statement is that comments are ignored by the Python interpreter and are not executed, whereas the pass statement is executed, but does not do anything.
Conclusion
In this article, we explored what does pass in Python and discussed its purpose and usage. We learned that the pass statement is used as a placeholder in incomplete code, and can be used in loops, functions, class definitions, and conditional statements. We also saw several examples that demonstrated the various use cases of the pass statement in Python.
Good Luck with your Learning !!
Related Topics:
1: Python Create Directory If It Doesn’t Exist
2: Fix Python Error: Legacy-Install-Failure
3: Random Word Generator Python
- How to Fix – TypeError: only size-1 arrays can be converted to Python scalars - 16 October 2023
- How to Implement d’wave qbsolv in Python - 16 October 2023
- Resolve Javascript error: ipython is not defined - 15 October 2023