Random Word Generator Python
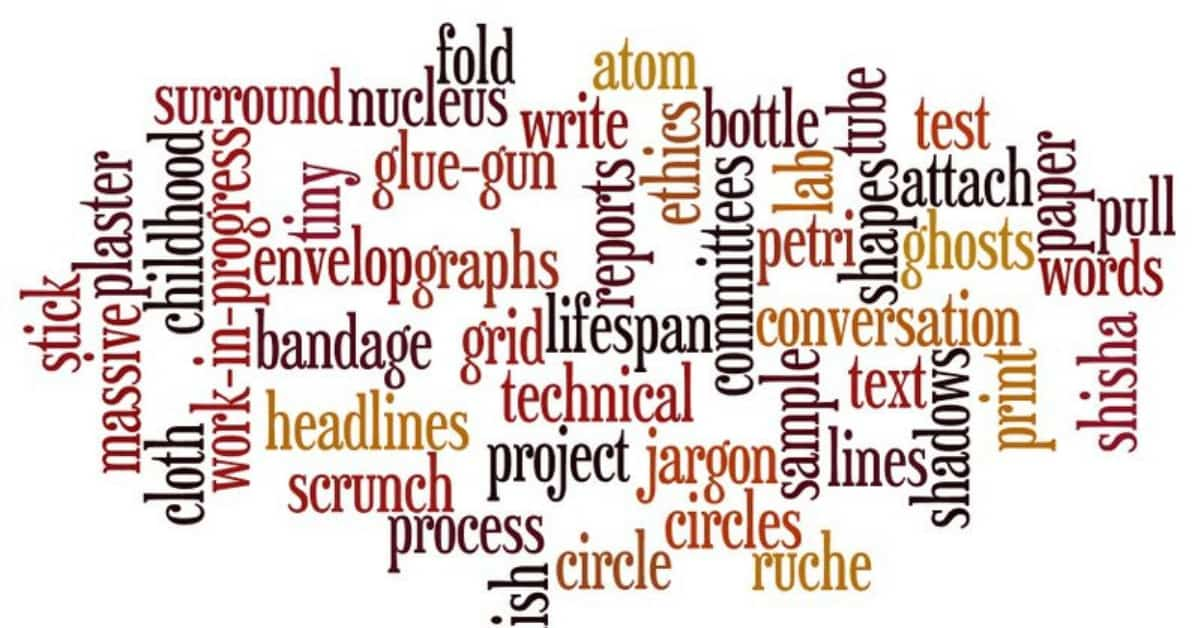
Today, we are going to use Python to build a “Random Word Generator Python” tool. This tool can create random words and can be used in a lot of areas like education, gaming, security, and more.
Read More: Things to Know Before Learning Python
Building a Robust Random Word Generator in Python
Python, known for its readability and efficiency, is the preferred choice for developers globally. In our endeavor to create a random word generator, we will leverage Python’s extensive libraries to randomize word lists and generate random words from both local and remote databases.
In this extensive guide, we provide a detailed walkthrough, enriched with real-world examples, tips, and code snippets to assist both new and seasoned developers in creating an optimized random word generator in Python.
Setting Up Your Python Environment
To kickstart your journey, it is imperative to have the Python environment set up and ready to go. If not, download Python from the official site and establish your development setting. Additionally, you will need the requests
module, which can be installed using the command:
pip install requests
Strategies to Generate Random Words
There are various strategies to build a random word generator. Here, we delve into two primary methods – utilizing a local file system and fetching data from a remote database via HTTP requests.
1: Randomize Word List: Utilizing a Local File System
In this method, we will utilize a local file containing a list of words to generate random words. The steps involved are simple and involve reading a file, manipulating strings, and using Python’s random module. Below are the detailed steps and a Python script exemplifying this method:
- Opening the File in Reading Mode: Utilize Python’s
open()
function to open your word file in reading mode. - Splitting the Content: Depending on how the words are structured in your file, use
str.splitlines()
orstr.split()
methods to split the content into a list of words. - Selecting a Random Word: Python’s
random.choice()
method comes in handy to select random words from the list created.
Here’s a Python script demonstrating this process:
import random
def get_list_of_words(path):
with open(path, 'r', encoding='utf-8') as f:
return f.read().splitlines()
# Replace with the path to your word list file
words = get_list_of_words('path/to/your/wordlist.txt')
random_word = random.choice(words)
print(random_word)
Exploring Further: Generating Multiple Random Words
Python’s prowess does not limit you to fetching a single random word. Leveraging list comprehension along with the random.choice()
method, you can easily fetch multiple random words. Here’s how:
n_random_words = [random.choice(words) for _ in range(3)]
print(n_random_words)
Real-World Applications and Examples
To further illustrate the utility of a random word generator in Python, let’s delve into its applications across various fields:
- Educational Apps: Apps that introduce learners to a new word daily, enhancing their vocabulary.
- Gaming: Popular games like Scrabble or Boggle where random words are needed to up the challenge.
- Creative Writing: Writers seeking random prompts can use this generator to fuel their creativity.
- Password Generators: In security systems, to formulate random and strong passwords.
- Quiz Applications: To spawn random questions from a curated list, ensuring a diverse question set in every quiz session.
2: Harnessing Remote Databases: Generating Random Words through HTTP Requests
Python’s capabilities stretch beyond local functionalities, empowering you to fetch data from remote databases. To generate random words from a remote database through HTTP requests, follow these steps:
- Initiating an HTTP Request: Make an HTTP request to a database hosting a word list using Python’s
requests
module. - Fetching and Processing the Data: Retrieve the data and process it to create a list of words.
- Random Word Selection: Use
random.choice()
the method to select random words from the list.
Here is the Python script showcasing this:
import random
import requests
def get_list_of_words():
response = requests.get('https://www.mit.edu/~ecprice/wordlist.10000', timeout=10)
return response.content.decode('utf-8').splitlines()
words = get_list_of_words()
random_word = random.choice(words)
print(random_word)
Diving Deep: Selecting Multiple Random Words
Similar to the local file method, you can select multiple random words using a list comprehension and the random.choice()
method. Here’s the script demonstrating this:
n_random_words = [random.choice(words) for _ in range(3)]
print(n_random_words)
Real-World Applications and Examples
Understanding the versatility of remote databases in generating random words, we find their applications in various domains:
- Content Creation Tools: Tools suggesting random keywords to include in blogs or articles to boost SEO.
- Language Learning Apps: Apps that generate random sentences from a database for learners to translate.
- E-commerce: E-commerce platforms recommend random products to users from a large database.
- Research: Academia and researchers leverage this tool to randomly select samples from a large dataset for various studies.
- Fitness Apps: Apps suggesting random workouts each day from a database of exercises.
Expert Tips and Best Practices
As you venture into creating a random word generator, here are some tips and best practices to keep in mind:
- File Handling: Ensure to handle files correctly, closing them after reading to avoid memory leaks.
- Error Handling: Implement error handling to manage potential errors gracefully, such as missing files or unsuccessful HTTP requests.
- Optimization: Optimize your code for better performance, avoiding redundant operations.
Frequently Asked Questions
How do I install the necessary Python modules for this tutorial?
Utilize pip install requests
to install the required modules.
Can I employ other databases to fetch the list of words?
Absolutely! You can use any database, ensuring it provides a list of words in a processable format in Python.
How can I guarantee the uniqueness of the generated random words?
To guarantee uniqueness, remove the chosen word from the list after each selection using the list.remove()
method.
What if the words in my file are divided by spaces instead of newlines?
Employ the str.split()
method without specifying any separator to divide the string at whitespace characters.
Conclusion
We have journeyed through the multifaceted process of creating a random word generator in Python, leveraging both local and remote databases. The applications of such generators are boundless, extending from enhancing learning experiences to securing systems with robust passwords.
Whether you are a budding developer keen on honing your Python skills or a seasoned coder looking to implement a random word generator in your next project, this guide offers you the resources to do so seamlessly.
As we part ways, we leave you with a tool enriched with potential, urging you to explore various databases and files to perfect your random word generator. Remember, the sky is the limit when you code with Python! Happy coding!
Read More:
Python Create Directory If It Doesn’t Exist
How to Find N Root of a Number – Python
AttributeError: ‘DataFrame’ object has no attribute ‘append’
- How to Fix – TypeError: only size-1 arrays can be converted to Python scalars - 16 October 2023
- How to Implement d’wave qbsolv in Python - 16 October 2023
- Resolve Javascript error: ipython is not defined - 15 October 2023