How to Implement d’wave qbsolv in Python
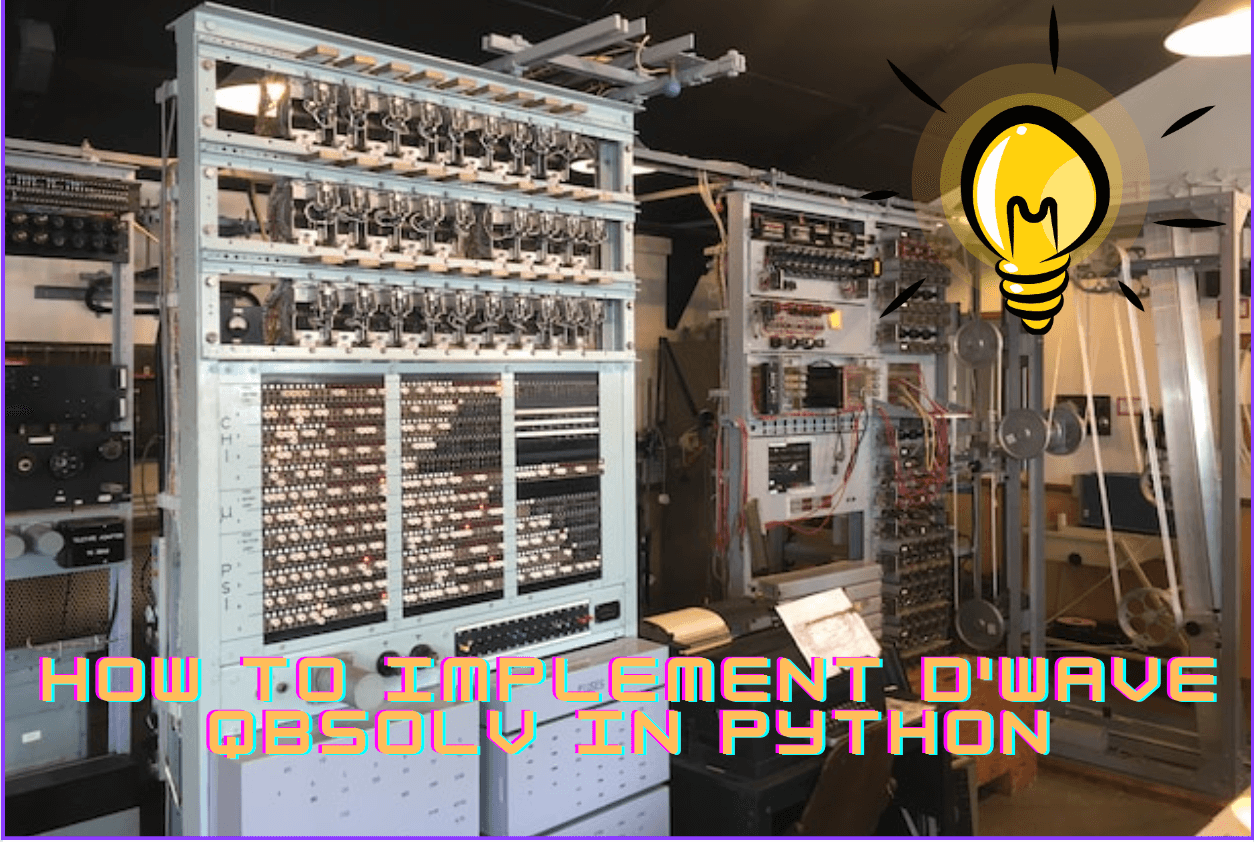
D-Wave’s quantum computer is capable of solving optimization problems in a fraction of the time it would take a classical computer. So what is D-Wave’s Qbsolv and how to implement it?
D-Wave’s QBSolv is a tool for solving Quadratic Binary Optimization (QBO) problems. QBSolv decomposes large-scale QUBO problems into smaller sub-problems that can be solved using quantum computing techniques. QBSolv is available as a Python library and has been used in a variety of applications
Introduction
In this article will explore how to implement QBSolv in Python, from installation to submitting problems to a D-Wave quantum computer for a solution. We will cover the various parameters and settings that can be used to fine-tune the performance of the solver. Whether you are a researcher, engineer, or data scientist, this guide will give you the knowledge and tools you need to harness the power of quantum computing for optimization.
Quantum computing is a rapidly growing field with the potential to revolutionize the way we solve complex problems. D-Wave’s quantum computer is one of the most powerful quantum computers in the world, capable of solving optimization problems in a fraction of the time it would take a classical computer.
D-Wave’s Qbsolv is a classical solver for binary quadratic models that uses quantum-inspired algorithms. It can be used to solve optimization problems. QBO problems are mathematical optimization problems that involve binary variables and quadratic constraints. They can be used to model many real-world optimization problems, for example, machine learning, logistics, and financial optimization.
Implement D-Wave’s QBSolv in Python
To implement D-Wave’s QBSolv in Python,
- Install the dwave-ocean-sdk library, which is the official Python library for the D-Wave quantum computer, using the following command
pip install dwave-ocean-sdk
- Sign up for an account on the D-Wave Leap cloud platform. To utilize D-Wave’s quantum computing systems, an account on the D-Wave Leap platform is required.
- Create a connection to the D-Wave quantum computer using the D-Wave Cloud Client:
from dwave.system.samplers import DWaveSampler
from dwave.system.composites import EmbeddingComposite
from dwave.cloud import Client
client = Client.from_config()
sampler = EmbeddingComposite(DWaveSampler())
- Define the optimization problem and convert the problem to a binary quadratic model (BQM).
import dimod
# Define the problem
Q = {(0, 0): 1, (0, 1): 2, (1, 1): 3}
# Convert the problem to binary quadratic model (BQM)
bqm = dimod.BinaryQuadraticModel.from_qubo(Q, offset=0.0)
- Solve the problem using the QBSolv solver as shown:
response = sampler.sample(bqm, num_reads=80, solver= 'QBSolv')
Note: We have Fine-tuned the solver’s performance by adjusting its parameters, num_reads in the above code. In the same way, you can change other parameters like chain_strength and post-process according to your needs.
- Retrieve the solution by calling the solver’s sample method which is in the form of dimod object here.
If you only want to extract the lowest energy sample, run this below command:
# Extract the solution
Lowest_sample_of_energy = response.first.sample
- If you want to extract all the samples with their corresponding energies, run the below command:
# Extract the solution
for sample, energy, num_occurrences in response.data(['sample', 'energy', 'num_occurrences']):
print(sample, energy, num_occurrences)
Note: To run this example, you need to have an active D-Wave account and API access to the D-Wave quantum computer.
Let’s consider another example below to understand the implement D-Wave’s QBSolv in Python:
# Load required libraries
import networkx as nx
import dimod
from dwave.system import DWaveSampler, EmbeddingComposite
from dwave.algorithms import qbsolv
# Create a random Ising model
G = nx.random_regular_graph(d=3, n=100)
J = {(u, v): 1.0 for (u, v) in G.edges()}
h = {v: 0.0 for v in G}
bqm = dimod.BinaryQuadraticModel.from_ising(h, J)
# Connect to a D-Wave quantum computer
sampler = EmbeddingComposite(DWaveSampler())
# Solve the problem using the qbsolv
response = qbsolv.sample_qubo(bqm, sampler)
# Get the samples from the solution
samples = response.samples()
for sample, energy, num_occurrences in response.data(['sample', 'energy', 'num_occurrences']):
print(sample, energy, num_occurrences)
This code will generate a random Ising model and then use QBSolv to solve it. The solution will be stored in the samples variable, a pandas data frame containing the binary assignments and their corresponding energies.
Explanation of the above code
The code imports the networkx and dimod libraries and uses them to generate a random Ising modeland solve it with D-Wave’s QBSolv algorithm.
- The first step is to create a random Ising model using the networkx library:
- The networkx.random_regular_graph function is used to generate a random regular graph with d edges per node and n nodes.
- The edges of the graph are stored in a dictionary J with the edge tuple as the key and the weight as the value.
- A dictionary h is created to store the biases for each node.
- The Ising model is then transformed into a QUBO (Quadratic Unconstrained Binary Optimization) problem using the dimod.BinaryQuadraticModel.from_ising function.
- The qbsolv.sample_qubo function is then used to solve the QUBO using D-Wave’s QBSolv algorithm. The result is stored in the response variable.
- The solution is stored in the samples variable, which is a pandas dataframe containing the binary assignments and their corresponding energies.
This code provides a basic example of how to use D-Wave’s QBSolv in Python to solve a random Ising model. We can be modified to solve other problems and use other features of the dwave-ocean-sdk library.
D-Wave’s quantum computer
D-Wave Systems Inc. has developed a quantum computer capable of solving optimization problems through quantum mechanics.
Unlike traditional computers, which utilize bits, this quantum device uses qubits that can exist in multiple states simultaneously, resulting in much quicker computation for specific types of problems.
D-Wave Ocean SDK
D-Wave Ocean SDK (Software Development Kit) is a collection of tools, libraries, and algorithms that D-Wave Systems Inc. provides for building and solving applications on their quantum computers which can be accessed using the Python Programming interface.
The Ocean SDK provides a Python library for solving optimization problems, including classical and quantum optimization solvers, as well as software tools for formulating problems, connecting to the quantum computer, and analyzing results. It also includes libraries and tools for using classical algorithms in conjunction with quantum computing and hybrid classical-quantum algorithms.
The Ocean SDK is designed to make it easier for developers, researchers, and scientists to take advantage of the computational capabilities of D-Wave’s quantum computers and to make it easier to solve complex problems in areas such as machine learning, quantum chemistry, and many others.
Note: The D-Wave Ocean SDK is also equipped with numerous examples and tutorials to aid users in their initial programming experience with quantum computers.
PyQUBO
PyQUBO is a Python library that enables the representation of QUBO (Quadratic Unconstrained Binary Optimization) and Ising problems as Python objects and their solution through either classical or quantum solvers.
It offers a clear and user-friendly API for expressing optimization problems and converts them into binary quadratic models that can be optimized using classical algorithms. The D-Wave quantum computer can find the global minimum of a potential energy function by transforming mathematical optimization into the Ising model and utilizing quantum annealing.
In other words, with PyQUBO, users can formulate QUBO problems in Python and use the D-Wave Ocean SDK to solve them on a D-Wave quantum computer. The library also supports the expression and solution of problems in the forms of Quadratic Binary Optimization (QBO) or Ising model. It provides the ability to convert between the three models.
Note: PyQUBO has multiple uses, including in fields such as machine learning, quantum chemistry, and quantum computing, and is compatible with well-known machine-learning frameworks like PyTorch and TensorFlow.
It makes it easier for developers and researchers to handle optimization problems and provides a seamless shift to quantum computing as it becomes more widely available.
QBSolv
QBSolv is a quantum binary solver developed by D-Wave Systems Inc. It is a software tool used to solve QUBO(Quadratic Binary Optimization) problems, which are mathematical optimization problems that can be mapped to the Ising model.
It is a classical optimization solver capable of handling optimization problems presented in either Ising model or QUBO format. This tool can be applied in various fields, such as machine learning, quantum computing, and quantum chemistry.
QBSolv is optimized for efficient computation and high performance on large-scale problems when run on classical computers. It is a component of the D-Wave Ocean SDK, which offers a complete environment for solving optimization problems, including QBSolv as one of the solvers specialized in solving QUBO problems.
Is D-Wave’s quantum computer capable of tackling issues other than optimization?
Yes, D-Wave’s quantum computer has the potential to solve a wide range of problems beyond optimization. It is primarily designed to solve optimization problems by mapping them to the Ising model and using quantum annealing to find the global minimum of a potential energy function. However, it can be used to solve other types of problems by mapping them to an Ising model, such as:
- Sampling problems: D-Wave’s quantum computer can be used to generate samples from a probability distribution. This can be useful for probabilistic machine-learning algorithms and quantum simulations.
- Constraint satisfaction problems: Some constraint satisfaction problems can be mapped to an Ising model and solved using D-Wave’s quantum computer.
- Machine Learning problems: D-Wave’s quantum computer can be used for unsupervised learning algorithms such as clustering and dimensionality reduction.
It can also be combined with classical computers and algorithms to solve more complex problems beyond the capability of quantum computing alone.
Is there an alternative library available for accessing and utilizing D-Wave’s quantum computer?
Yes, there are alternative libraries available for accessing and utilizing D-Wave’s quantum computer. Some of the commonly used libraries include:
- PyQUBO: a Python library for expressing and solving Quadratic Unconstrained Binary Optimization (QUBO) problems.
- Dwave-system: a library for constructing and solving problems on the D-Wave system.
- NetworkX: a Python library for the creation, manipulation, and study of the structure, dynamics, and functions of complex networks.
- Dimod: a Python library for building and solving Ising and quadratic unconstrained binary optimization (QUBO) problems. It is designed to be used with quantum annealing-based optimization algorithms.
Each library has its own unique features and capabilities, and it’s essential to choose the right one based on the specific requirements of the problem you’re trying to solve and your own programming expertise.
Conclusion
In this article, we saw how to use D-Wave’s Qbsolv in Python to solve binary quadratic models(BQMs). We used the dimod
library, which provides a unified interface to various classical and quantum optimization solvers, including Qbsolv, and can easily and efficiently solve BQMs and find the configuration of binary variables that minimize the energy of the system.
Good Luck with your Learning!!
Related Topics:
Python Program to Find Armstrong Numbers in an Interval
What datatype is represented by int in python 3
How to send JSON data in python flask
Coding Spell | Python Journey | About Us
- How to Fix – TypeError: only size-1 arrays can be converted to Python scalars - 16 October 2023
- How to Implement d’wave qbsolv in Python - 16 October 2023
- Resolve Javascript error: ipython is not defined - 15 October 2023