How to Check if a Variable is Null in Python
Checking if a Variable is Null is a very common task in python, In this article, we’ll walk you through the process step-by-step and provide real-time examples to help you understand how it works. Let’s dive in!
To check if a variable is null in Python, you can use the “is” keyword or the “==” operator. The “is” keyword is more efficient when checking for null values. You can also use the .get()
method to check if a key in a dictionary is null and assign a default value if it is. Checking for null variables is important to avoid errors in your program.
Introduction
Python is a high-level programming language that is widely used in various fields, including web development, data science, machine learning, and more. In Python, variables are used to store values such as numbers, strings, and lists. Sometimes, these variables may have null values, which means that they don’t have any value assigned to them. In this article, we’ll discuss how to check if a variable is null in Python.
What is a Null Variable?
A null variable is a variable that doesn’t have any value assigned to it. In Python, null values are represented by the keyword “None”. When a variable is assigned to None, it means that the variable has no value. Checking if a variable is null is important in programming because it allows you to handle cases where variables are not assigned any values.
How to Check if a Variable is Null in Python
In Python, you can check if a variable is null by using the “is” keyword or the “==” operator. The “is” keyword checks if two variables refer to the same object, while the “==” operator checks if two variables have the same value.
To check if a variable is null using the “is” keyword, you can use the following syntax:
if variable is None:
# code to execute if variable is null
To check if a variable is null using the “==” operator, you can use the following syntax:
if variable == None:
# code to execute if variable is null
NOTE: “is” keyword is faster and more efficient than the “==” operator when checking for null values.
Examples of Checking for Null Variables in Python
Here are some examples of checking for null variables in Python:
Example 1: Using the “is” Keyword
# check if variable is null using the "is" keyword
var = None
if var is None:
print("Variable is null")
Output:
Variable is null
Example 2: Using the “==” Operator
# check if variable is null using the "==" operator
var = None
if var == None:
print("Variable is null")
Output:
Variable is null
Example 3: Checking if a Variable is not Null
# check if variable is not null
my_var = "Hello, world!"
if my_var is not None:
print("my_var is not null")
Output:
Variable is not null
Real-time example:
We are trying to check if the “position” key in their dictionary is null, If yes, we will replace it with “Unknown” in the below dictionary
Example:
employee_info = { "Tom": {"age": 25, "position": "Engineer", "salary": 50000}, "Jen": {"age": 30, "salary": 60000}, "Richie": {"age": 35, "position": "Manager", "salary": 80000} } print(employee_info) # check if each employee has a position assigned, and assign a default value if not for employee in employee_info: if employee_info[employee].get("position") is None: employee_info[employee]["position"] = "Unknown" print(employee_info)
Output:
Before
{‘Tom’: {‘age’: 25, ‘position’: ‘Engineer’, ‘salary’: 50000}, ‘Jen’: {‘age’: 30, ‘salary’: 60000}, ‘Richie’: {‘age’: 35, ‘position’: ‘Manager’, ‘salary’: 80000}}
After
{‘Tom’: {‘age’: 25, ‘position’: ‘Engineer’, ‘salary’: 50000}, ‘Jen’: {‘age’: 30, ‘salary’: 60000, ‘position’: ‘Unknown’}, ‘Richie’: {‘age’: 35, ‘position’: ‘Manager’, ‘salary’: 80000}}
Process finished with exit code 0
In this example, we loop through each employee in the dictionary and use the .get()
method to check if the “position” key is null. If it is, we assign a default value of “Unknown” to that key. This ensures that every employee in the dictionary has a value for the “position” key, even if they haven’t been assigned a position yet.
Reference:
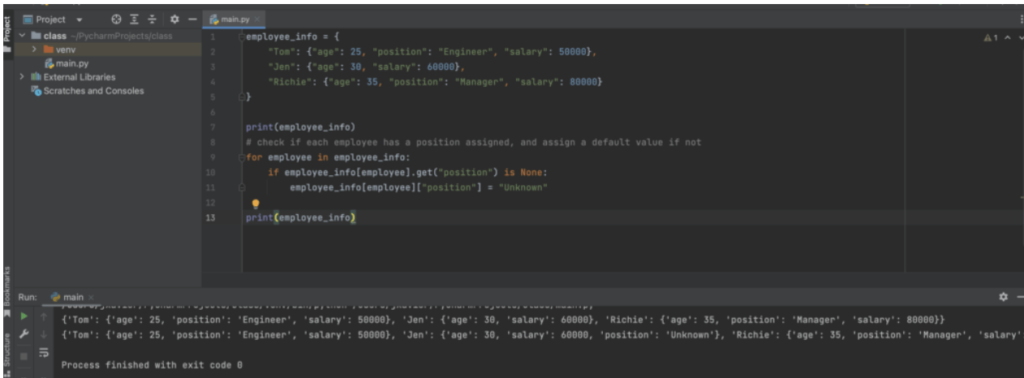
Conclusion
Checking if a variable is null is an important part of programming in Python. In this article, we discussed how to check if a variable is null using the “is” keyword and the “==” operator. We also provided examples of checking for null variables in Python. By understanding how to check for null variables, you’ll be able to write more robust and error-free Python code.
Good Luck with Your Learning !!
Related Topics:
How do I reinstall Anaconda Python
How to convert RGB to Hex and Hex to RGB code in Python
What is the difference between print and return in Python?
Coding Spell | Python Journey | About Us
- How To Find the Length of a List in Python - 16 October 2023
- Python Program to Swap Two Variables - 16 October 2023
- How to Check if a Variable is Null in Python - 14 October 2023