How to convert RGB to Hex and Hex to RGB code in Python
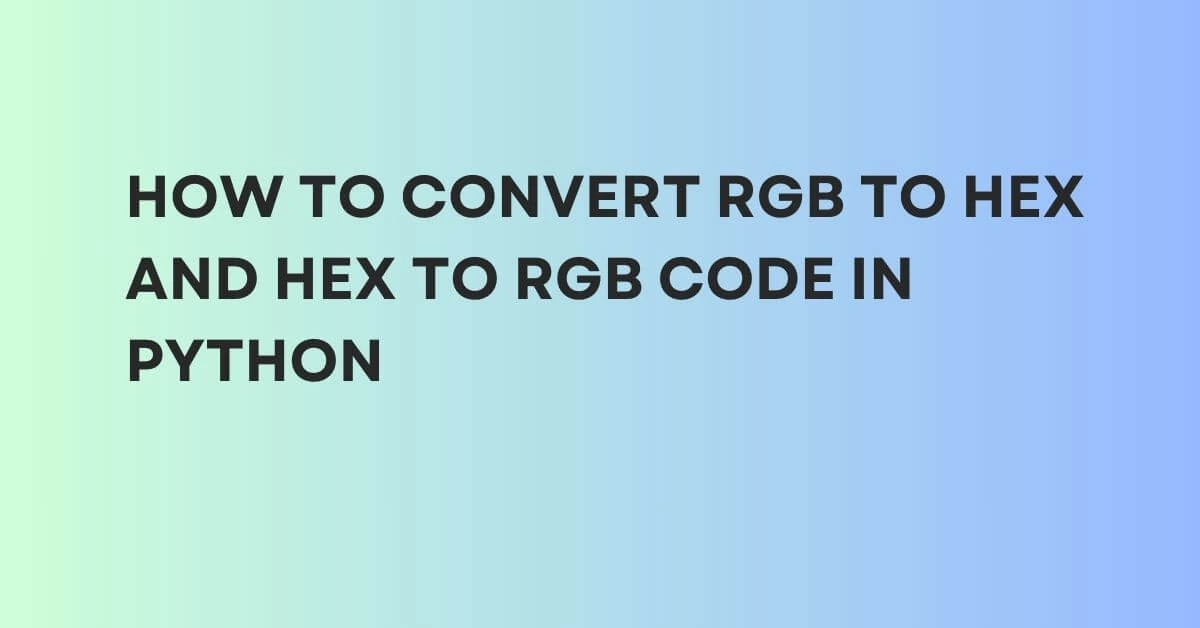
Color representation is an essential part of various digital applications, including web design, graphic design, and image processing. RGB and hex codes are color models used to represent colors digitally, Knowing how to convert between them would be helpful for Web designers and developers. In this blog, we will learn about the basics of RGB and Hex code, and how to convert between them using Python
To convert RGB to hex and hex to RGB code in Python, use the hex() and int() functions along with three arguments for the red, green, and blue values.
RGB to hex -> f"#{hex(red)[2:]}{hex(green)[2:]}{hex(blue)[2:]}"
hex to RGB -> tuple(int(hex_code[i:i+2], 16) for i in (1, 3, 5))
Before, going further, let’s understand hex and RGB in detail

What is Hex code
Hex code -> hexadecimal code is a color model used to represent colors in web design, graphic design, and image processing. It is a six-digit code that consists of a pound sign (#) followed by a combination of six letters and numbers
Example: #ff0000 -> Red Colour
Out of 6 digits, each pair of digits in the hex code represents the intensity of one of the three primary colors: red, green, and blue (RGB). The first two digits denote the red intensity, the second two digits denote the green intensity, and the last two digits denote the blue intensity (Shown in the table below).
The range of intensity is from 00(low) -> ff(high). Taking the above example #ff0000
ff | 00 | 00 | Result |
Red (high intensity) | Green(No Intensity) | Blue (No intensity) | Red Colour |
NOTE: Based on the intensity, your end results may vary
What is RGB code
RGB code is a color model used to represent colors in various digital applications as same as hex. RGB stands for R-> Red, G-> Green, and B->Blue, These are the primary colors used to create all other colors.
Example: (0, 255, 0) -> Green Colour
RGB is represented as a combination of the intensity values of these three primary colors (Red, Green, and Blue). As shown in the table below
The range of intensity is from 00(low) -> 255(high). Taking the above example (0, 255, 0)
0 | 255 | 0 | Result |
Red(No intensity) | Green(High intensity) | Blue(No intensity) | Green Colour |
RGB code is easy to use and understand, as it directly represents the intensity values of the three primary colors.
Convert RGB to Hex Code
To convert RGB to hex code in Python, We have created a custom function called rgb_to_hex, Which takes an RGB color tuple (e.g. (255, 0, 0)
) as input and returns a hex color code (e.g. "#ff0000"
).
def rgb_to_hex(rgb):
#Converts an RGB color => hex color code.
r, g, b = rgb
return "#{:02x}{:02x}{:02x}".format(r, g, b)
#Calling the function
print(rgb_to_hex((0, 0, 255)))
Output:
'#0000ff'
In the above example, we have passed the RGB tuple (0, 0, 255)
to the rgb_to_hex
function, which returned the corresponding hex code =>'#
=> Red Colour.0000ff
'
Convert Hex to RGB
To convert hex to RGB in Python, We have created a custom function called hex_to_rgb, Which takes a hex color code string (e.g. "#ff0000"
) as input and returns an RGB color tuple (e.g. (255, 0, 0)
).
def hex_to_rgb(hex_code):
#Converts a hex color code => RGB color.
hex_code = hex_code.lstrip('#')
return tuple(int(hex_code[i:i+2], 16) for i in (0, 2, 4))
#Calling the function
print(hex_to_rgb('#ff0000'))
Output:
(255, 0, 0)
In the example above, we passed the hex code '#ff0000'
to the hex_to_rgb
function, which returned the corresponding RGB tuple (255, 0, 0)
.
Use of this Conversion (RGB -> HEX and HEX -> RGB)
The conversion between RGB and hex code is useful in various digital applications
For example, in web design and development, CSS (Cascading Style Sheets) often uses hex code to specify colors for web pages. By converting RGB to hex code, Developers can easily specify colors for their web pages using a six-digit code(#ff0000). Similarly, in graphic design, hex code is commonly used to specify colors for logos, icons, and other design elements.
On the other hand, some image processing libraries and tools may use RGB code to represent colors. By converting hex code to RGB code, developers can work with color data in a format that is compatible with their image processing tools.
As discussed, The conversion between RGB -> hex and hex -> RGB is useful for working with colors in digital applications. It helps developers to easily convert between each other and work with the color data in a format that is most suitable for their application.
Conclusion
In Conclusion, We have learned to convert RGB to hex code and hex to RGB in Python. These conversions will be useful when working with colors, such as web development, data visualization, image processing, etc.
Read More:
How to find the length of a string in Python (With wordcount example)
Fix: Ray Out Of Memory Error Python
Fix – TypeError: tuple indices must be integers or slices not str
How to Make Jarvis With Dialogflow and Python
Coding Spell | Python Journey | About Us
- How To Find the Length of a List in Python - 16 October 2023
- Python Program to Swap Two Variables - 16 October 2023
- How to Check if a Variable is Null in Python - 14 October 2023