Fix – TypeError: tuple indices must be integers or slices not str
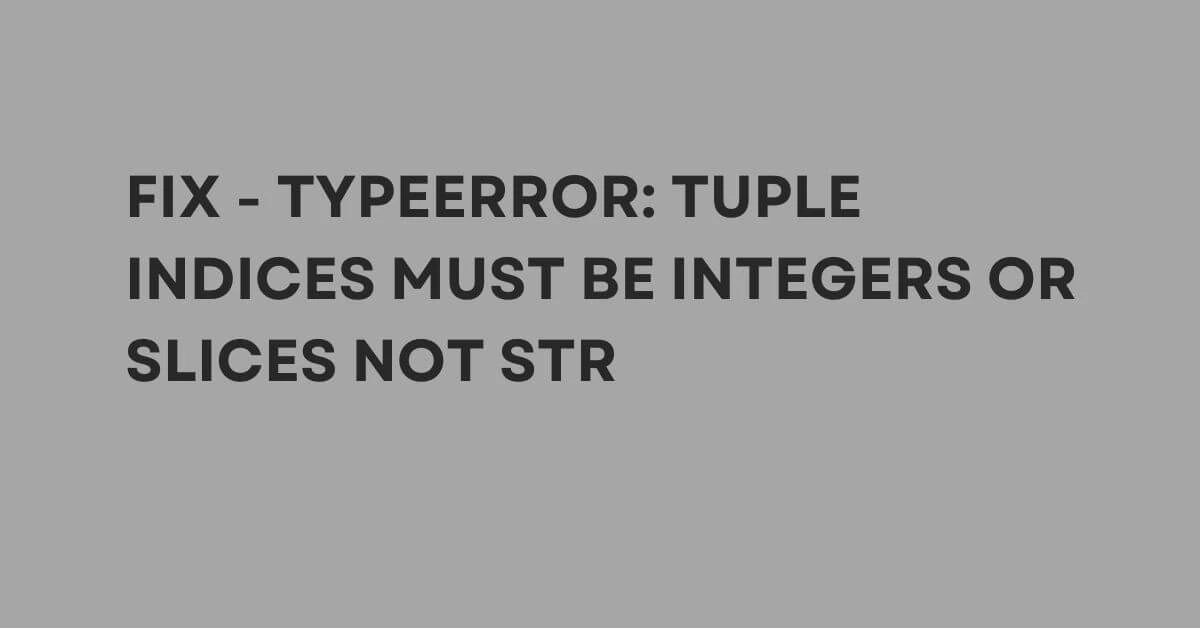
This TypeError: tuple indices must be integers or slices not str error usually occurs when we try to access the element of the tuple using a string as the index. In this article, we will explain the cause of this error and also, and we will see different solutions to fix it.

What is a tuple in Python?
A tuple is a collection of ordered and immutable elements in Python. It is similar to a list, but once created, its elements cannot be changed.
To create a tuple, place a sequence of elements between parentheses, as follows:
myTuple = (1, 2, 3, "hello", True)
Accessing elements of a tuple
We can access the elements of a tuple by using indexing. Here is an example:
myTuple = (1, 2, 3, "hello", True) print(myTuple[0]) print(myTuple[3]) print(myTuple[-1])
Output
1
hello
True
In the above code snippet, 1 is the first element, hello is the third, and True is the last element of the tuple. It is important to note that the index of the first element in a tuple is 0, and the index of the last element is – 1 as shown above.
What causes the TypeError: tuple indices must be integers or slices not str error and How to Fix it?
This error can occur due to different reasons few of which are as follows:
Using a String as an Index to a Tuple
This error occurs when we try to access an element of a tuple using a string as the index. For example:
myTuple_2 = ('apple', 'banana', 'cherry', 'mango')
index = '1'
print(myTuple_2[index])
In this code, the index variable contained a string value ‘1‘ to access the element of myTuple_2 which will cause the TypeError: tuple indices must be integers or slices not str error.
Have a look at another example that can cause this error:
myTuple_2 = ('apple', 'banana', 'cherry', 'mango') user_input = input("Enter an index to access an element of the tuple: ") index = user_input print(myTuple_2[index])
Output
TypeError: tuple indices must be integers or slices, not str
Here, the input() function is used to get user input for an index to access an element of a tuple. The input that we take from the user is a string value which is the reason behind the above error.
Solution
To fix the TypeError: tuple indices must be integers or slices not str error, use the following solutions.
1. Use an Integer as an Index
To access an element of a tuple, use an integer as an Index to avoid the above error. As shown below:
myTuple_2 = ('apple', 'banana', 'cherry', 'mango') print(myTuple_2[1])
Output
banana
In this example, the element of myTuple_2 is accessed using an integer index. Here, 1 is representing the second element of the tuple.
Here’s an example of how to handle user input that may contain strings instead of integers:
myTuple_2 = ('apple', 'banana', 'cherry', 'mango') user_input = input("Enter an index to access an element of the tuple: ") index = int(user_input) print(myTuple_2[index])
Output
Enter an index to access an element of the tuple: 2
cherry
In this example, the input() function is used to get user input for an index to access an element of a tuple. Since user input is typically a string, the int() function is used to convert the input to an integer. By using the int() function, we can avoid the TypeError: tuple indices must be integers or slices not str error
2. Use Slicing as an Index
To access an element of a tuple, use slicing as an Index to avoid the above error.
Consider the below example to understand how to use slicing:
myTuple_2 = ('apple', 'banana', 'cherry', 'mango')
print(myTuple_2[0:2])
Output
('apple', 'banana')
Here, the first two elements of myTuple_2 are accessed using slicing myTuple_2[0:2]. Here, we wanted to extract elements starting from index 0 up to index 2.
Note: Element at ending index of slicing (e.g, 2) is not included in the output.
3. Convert String variable to Integers
Another solution to avoid the TypeError: tuple indices must be integers or slices not str error is to convert the string variable taken from user input to integers. Here’s an example:
myTuple_2 = ('apple', 'banana', 'cherry', 'mango') index = '1' print(myTuple_2[int(index)])
Output
banana
In this example, the index variable is a string containing the value ‘1’. The int() function is used to convert the index variable to an integer. Then, the resulting integer value is used as the index to access the second element of the tuple, which is a banana.
Typos or Incorrect Variable Names
Typos or incorrect variable names can also lead to this error. Therefore, always correct the typo or use the correct variable name to prevent the error.
Conclusion
In conclusion, the TypeError: tuple indices must be integers or slices not str error occurs when you try to use a string as the index to a tuple or in other words, pass a string as the index to a tuple. This error can also occur due to typos, or incorrect variable names. To fix this error, make sure to use an integer or slice as the index and double-check your code for any mistakes. Additionally, to work with user input or data from an external source that may contain strings instead of integers, we can avoid this error by converting any string values to integers before using them as indices.
Read More:
What is the difference between print and return in Python?
How to find the length of a string in Python (With wordcount example)
Fix: Ray Out Of Memory Error Python
How to convert RGB to Hex and Hex to RGB code in Python
Coding Spell | Python Journey | About Us
- How To Find the Length of a List in Python - 16 October 2023
- Python Program to Swap Two Variables - 16 October 2023
- How to Check if a Variable is Null in Python - 14 October 2023