What is the difference between print and return in Python?
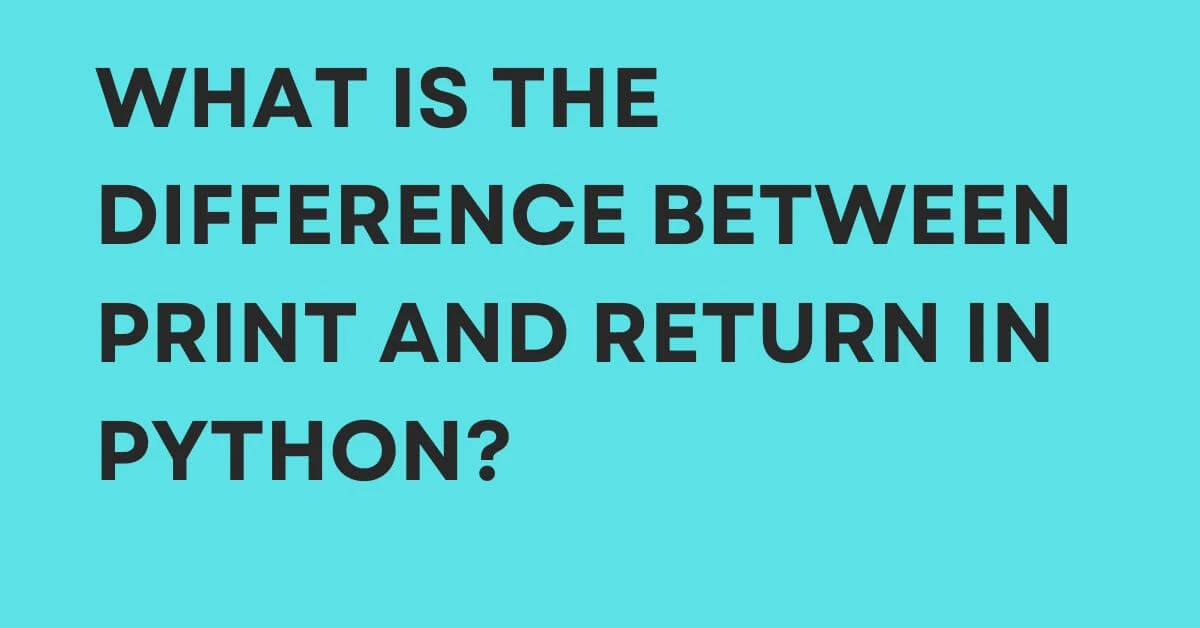
Print takes the values/variables as input and displays the value in the console whereas the return keyword is used in functions to give back the value to the place where the function is called and will exit the function.
Example:
Print:
print ("Learn & Share") a="Learn & Share" print a," & ", a
Output:
Learn & Share Learn & Share & Learn & Share
In the above example, the Print function gets the string as an argument and prints it back into the console
Return:
#defining a function def add(num1, num2): return num1+num2 print("checking") #Calling a function answer=add(10, 100) print(answer)
Output:
110
In the above example, the return keyword will exit the function, which will not allow the line “print(“checking”)” to be executed
Where to use print:
As mentioned above, the print function will take a variable or value as an input and print the output in the console. Usually helpful, When you want to see the result up front in the console, Also, As part of debugging to verify if the value getting assigned is valid or not
Print output cant be used anywhere else in the program, It will just show the output
Where to use return:
The return keyword is used in the function to return the value instead of printing it in the console, This will be more flexible in case of assigning the function output to a variable or feeding it as an input to other functions.
Example of where return would be more efficient:
Check this simple calculator program:
#Calculator def add(n1, n2): return n1 + n2 def subtract(n1, n2): return n1 - n2 def multiply(n1, n2): return n1 * n2 def divide(n1, n2): return n1 / n2 #Program has been trimmed to just explain the return usage num1= 100 num2= 100 num3=500 answer = multiply(add(num1, num2), num3) print(answer)
Output:
100000
In the above example, With the return keyword, We can just directly use the output to a different function. In this way, It will save a lot of code ๐
Quick tips:
Using just the return keyword, without any value or variable will give you “none”
def my_function(): return print(my_function())
Output:
None
Final thoughts:
It is important to understand the difference between print and return keyword and use it where it meant to be used
Printing is to show the value in the console and can’t be reused
Return is to return the value, where the function is called and can be reused.
Read More:
TypeError: method() takes 1 positional argument but 2 were given
TypeError: list indices must be integers or slices not tuple
Fix โ ImportError: cannot import name force_text from django.utils.encoding
How to find the length of a string in Python (With wordcount example)
Coding Spell | Python Journey | About Us
- How To Find the Length of a List in Python - 16 October 2023
- Python Program to Swap Two Variables - 16 October 2023
- How to Check if a Variable is Null in Python - 14 October 2023