TypeError: method() takes 1 positional argument but 2 were given

Hey there! Have you ever encountered the “TypeError: Person.say_hello() takes 1 positional argument but 2 were given” error in Python?
The “TypeError: method() takes 1 positional argument but 2 were given” error in Python is caused by passing too many arguments to a function or method that only expects one. To fix this error, one needs to ensure the correct number of arguments are passed when calling the method, as per its documentation. Ignoring this error can lead to program failure and unexpected behavior.
Introduction
Python is an object-oriented programming language. In Python, functions and methods are defined with a specific number of arguments. When a method is called with a different number of arguments than it expects, Python raises a TypeError, which can cause your program to crash.
One common TypeError is “TypeError: method() takes 1 positional argument but 2 were given.” This error occurs when a method is called with too many arguments. In this article, we will explore this error and how to fix it.
What is “TypeError: method() takes 1 positional argument but 2 were given”?
When Python raises “TypeError: method() takes 1 positional argument but 2 were given,” it means that a method has been called with more arguments than it expects. A method is a function that is associated with an object, and is defined with a certain number of arguments. If a method is called with more arguments than it expects, Python raises a TypeError.
Reason 1:
This error typically occurs when a method is defined to take a specific number of arguments but is called with a different number of arguments. In the case of the “TypeError: Person.say_hello() takes 1 positional argument but 2 were given” error, the say_hello() method of the Person class is defined to take one argument, but it is being called with two arguments.
Example:
class Person: def say_hello(name): print(f"Hello, {name}!") Person.say_hello("Learn","Share")
Output:
TypeError: Person.say_hello() takes 1 positional argument but 2 were given Process finished with exit code 1
Reference:

In this code, we have defined a Person class with a say_hello() method that takes one argument, name. We then create a Person object and call the say_hello() method with two arguments, “Alice” and “Bob”. This will result in the “TypeError: Person.say_hello() takes 1 positional argument but 2 were given” error because the say_hello() method only expects one argument.
Solution
To fix this error, we need to make sure that we are calling the say_hello() method with the correct number of arguments. In the case of the Person class, we need to make sure that we are only passing one argument to the say_hello() method.
Example:
class Person: def say_hello(name): print(f"Hello, {name}!") Person.say_hello("Learn")
Output:
Hello, Learn! Process finished with exit code 0
Reference:
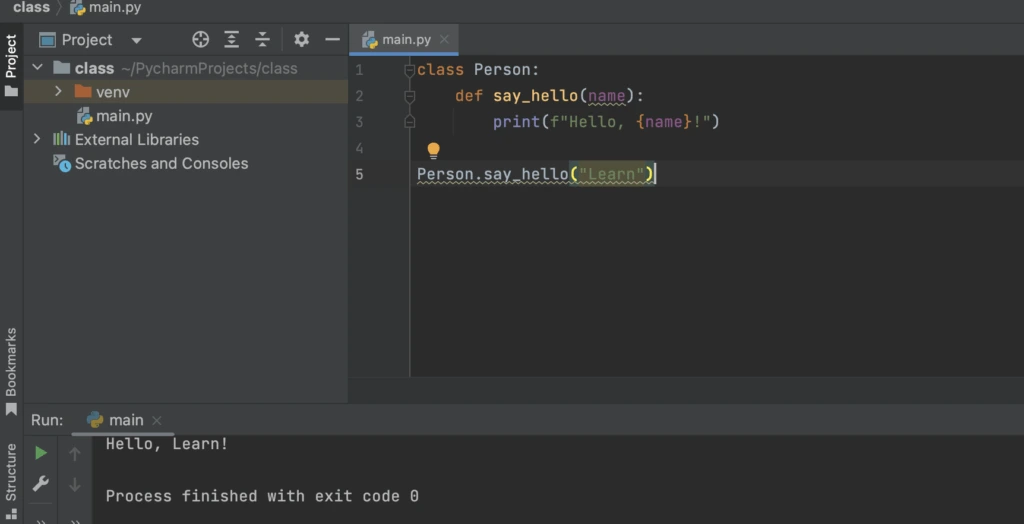
n this modified code, we have removed the second argument from the say_hello() method call, so it is only passed one argument. Now when we run this code, the say_hello() method will execute without any errors.
Reason 2:
Error message occurs when you call a method with an instance of a class, but forget to include the “self” parameter, That is you are passing 1 argument + instance of a class as below
Example:
class Person: def say_hello(name): print(f"Hello, {name}!") person = Person() person.say_hello("Learn")
Output:
TypeError: Person.say_hello() takes 1 positional argument but 2 were given Process finished with exit code 1
In this example, we have defined a Person class with a say_hello() method that takes one argument, name. However, when we call the say_hello method on the Person class using an instance of the class, we get the “TypeError: Person.say_hello() takes 1 positional argument but 2 were given“
Solution
To fix this error, We need to make sure that we are only passing one argument to the say_hello() method and either include the “self” parameter or access the class without creating the instance
Example: including the self parameter in the method
class Person:
def say_hello(self,name):
print(f"Hello, {name}!")
person = Person()
person.say_hello("Learn")
Example: Accessing the class without creating an instance
class Person: def say_hello(name): print(f"Hello, {name}!") Person.say_hello("Learn") Output: Hello, Learn!
Reference:

Note: In case, You are seeing a different error like “TypeError: Person.learn() missing 1 required positional argument: ‘self'”
It is because, If we are passing one argument without creating an instance of a call but we have included the self argument in the methods then will get a different error, Which is covered in this article “TypeError: Person.learn() missing 1 required positional argument: ‘self'”
Example: class Person: def say_hello(self, name): print(f"Hello, {name}!") Person.say_hello("Learn")
Conclusion
In conclusion, the “TypeError: Person.say_hello() takes 1 positional argument but 2 were given” error is a common error that developers encounter when calling a method with an incorrect number of arguments. To fix this error, we need to make sure that we are calling the method with the correct number of arguments that it expects.
Read More:
Fix – attributeerror: module numpy has no attribute int
FIX – TypeError: list indices must be integers or slices not float
How to Send SMS Text Messages Using Python
TypeError: list indices must be integers or slices not tuple
Coding Spell | Python Journey | About Us
- How To Find the Length of a List in Python - 16 October 2023
- Python Program to Swap Two Variables - 16 October 2023
- How to Check if a Variable is Null in Python - 14 October 2023