How to Send SMS Text Messages Using Python
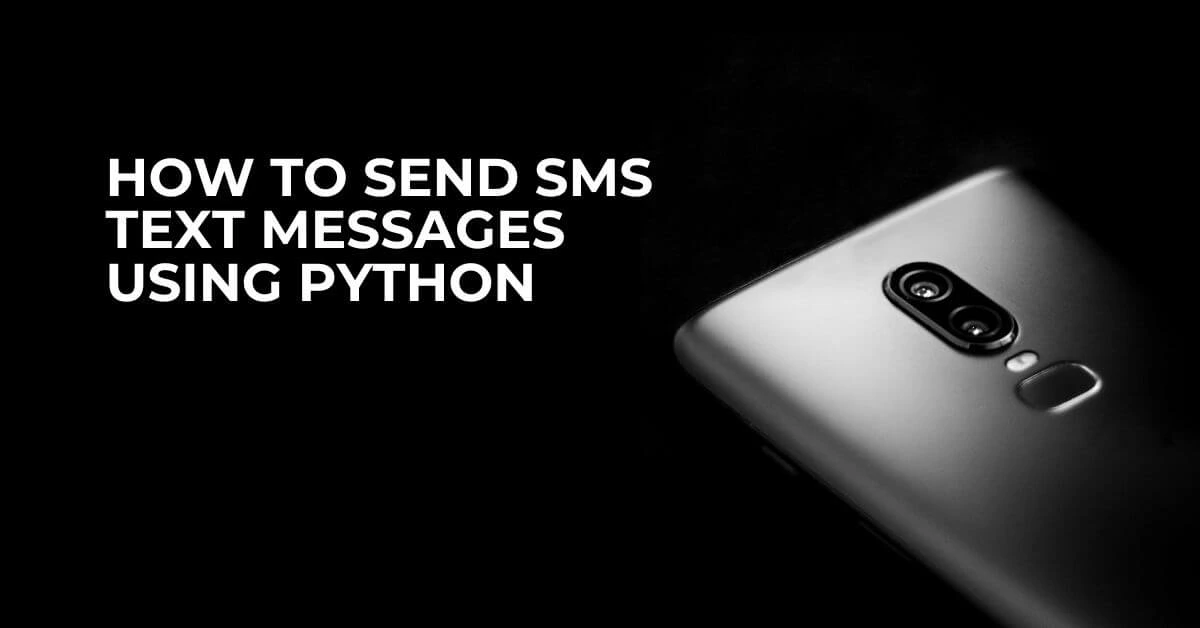
If you are a system administrator who needs to send alert SMS messages to multiple users based on filesystem usage or recent activities in the system, this article is for you. To Send SMS Text messages from Python can be useful in various scenarios such as sending notifications, alerts, or reminders to users. In this article, we will explore how to send SMS messages using Python.

There are multiple vendors like Twilio, and Fast2SMS exposing their API to send SMS, Out of all, We are going to have a discussion about Fast2SMS API and how to send SMS using this.
Prerequisites – To Send SMS Text Messages
Before you begin, you will need the following:
- Create a Fast2SMS account
- A phone number that can receive SMS messages (This is for testing)
- Python 3.6 or higher installed on your computer
- The
requests
Python package installed (If it is not installed, Its ok, We are sharing the steps in the article)
Setting up your Fast2SMS account
If you haven’t already, sign up for a Fast2SMS account at fast2sms.com. Once you have signed up and verified your account, you’ll need to obtain your Fast2SMS API key, which you can find in your Fast2SMS account dashboard.
NOTE: its a paid service, you need to at least recharge for 2$ to send your 1st SMS π and it’s the same for other service providers as well.

NOTE: In case you are using Twilio API in Python, you’ll need to install the twilio
package. The remaining steps would be as same as those below
Installing the requests package
We need to install the requests
package to use Fast2SMS API. You can do this using pip, the package installer for Python. Open a command prompt or terminal window and enter the following command:
pip install requests
If you are using Pycharm for this exercise, Follow the below steps to install “requests” package
Pycharm -> preferences -> Python Interpreter -> click ‘+’ icon -> Search package “requests” -> Install

Set up your Python script
As we have completed all the pre-requisite setups, Now We are ready to send an SMS message from Python. We will Start by opening a new Python file in your terminal or favorite code editor or IDE (I am using Pycharm to execute this code). Define the following variables at the top of your file:
import requests #Your Fast2SMS API key api_key = 'your_api_key' #phone number of Sender from_number = '9876543xxx' #phone number of Receiver to_number = '1234567890' #Actual message to send message = 'Hello everyone, Learn & Share is a place where we can collaborate and expand our knowledge together.'
In the above example code, replace
- your_api_key with your Fast2SMS API key
- from_number with the Sender’s phone number
to_number
with Receiver’s phone number- message with the Actual message you wanted to send
Now, We have declared all the variable in the python script, Once done will move into the next step of defining API endpoint
Define the API endpoint and payload
url = 'https://www.fast2sms.com/dev/bulkV2' # The payload to send with the request payload = { 'sender_id': 'FSTSMS', 'message': message, 'language': 'english', 'route': 'p', 'numbers': to_number } # The headers to send with the request headers = { 'authorization': api_key, 'Content-Type': "application/x-www-form-urlencoded", 'Cache-Control': "no-cache" }
In the above code, url
is the Fast2SMS API endpoint for sending SMS messages. payload
is a dictionary containing the parameters to send with the request which includes the sender ID, message content, language, message route, and receiver phone number. headers
is a dictionary containing the authorization token and other headers to send with the request.
Send the SMS message
# Send the SMS message response = requests.post(url, data=payload, headers=headers) # Check the response status code if response.status_code == 200: print('SMS message sent successfully!') else: print('Failed to send SMS message.')
In the above code, we use the requests.post()
method to send a POST request to the Fast2SMS API endpoint with all the defined payload
and headers
variables. The response from the API call is stored in the response
variable. We then check the response status code to determine if the message was sent successfully (status code 200 means successful else failure).
Final Complete Code
import requests api_key = 'Your API Key' # phone number of Sender from_number = '9994899101' # phone number of Receiver to_number = '9791874202' # The message you want to send message = 'Hello everyone, Learn & Share is a place where we can collaborate and expand our knowledge together.' url = 'https://www.fast2sms.com/dev/bulkV2' # The payload to send with the request payload = { 'sender_id': 'FSTSMS', 'message': message, 'language': 'english', 'route': 'p', 'numbers': to_number } # The headers to send with the request headers = { 'authorization': api_key, 'Content-Type': "application/x-www-form-urlencoded", 'Cache-Control': "no-cache" } # Send the SMS message response = requests.post(url, data=payload, headers=headers) # Check the response status code if response.status_code == 200: print('SMS message sent successfully!') else: print('Failed to send SMS message.')
Run the script
If you are running this in the terminal, save the same with a name like fast2sms.py
. Then, run the script using the following command or from the IDE
python fast2sms.py
Once everything done, you should be seeing a message indicating that the SMS message was sent successfully! else you will be seeing failed to send SMS
Output:
SMS message sent successfully! Process finished with exit code 0
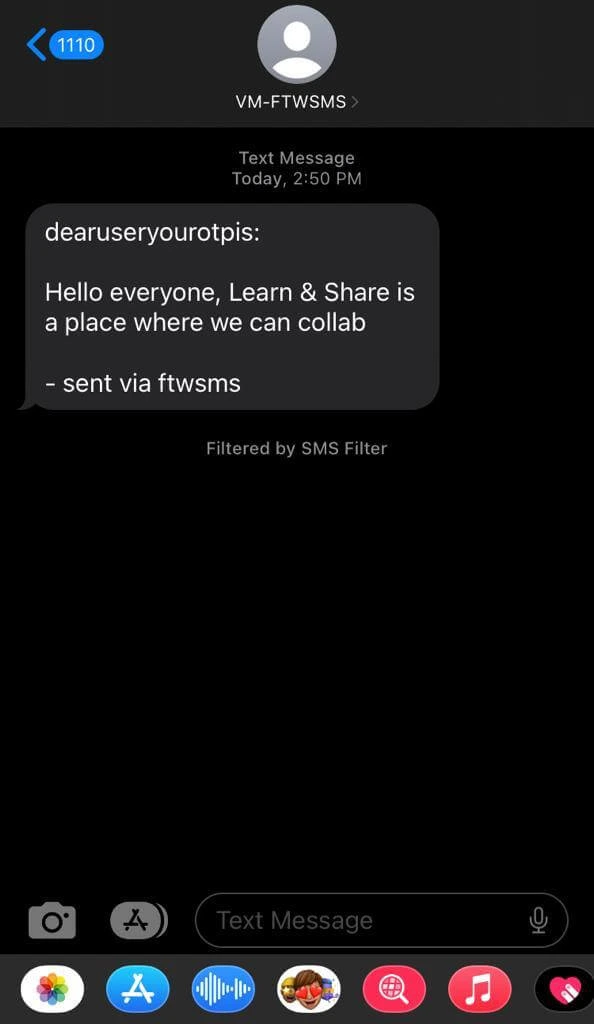
Conclusion
In this article, we walked through the process of sending an SMS message from Python using the Fast2SMS API. By following these steps, you can easily integrate SMS messaging into your Python projects.
Read More:
Fix β attributeerror: module numpy has no attribute int
FIX β TypeError: list indices must be integers or slices not float
TypeError: method() takes 1 positional argument but 2 were given
Coding Spell | Python Journey | About Us
- How To Find the Length of a List in Python - 16 October 2023
- Python Program to Swap Two Variables - 16 October 2023
- How to Check if a Variable is Null in Python - 14 October 2023