How to Convert JSON to YAML
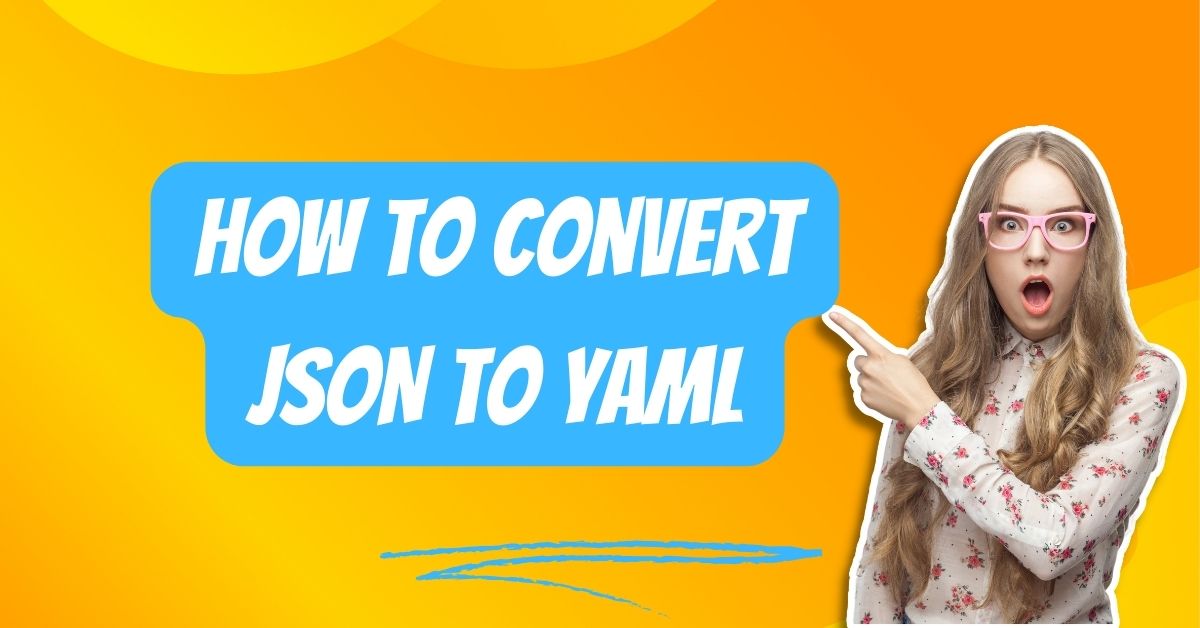
In today’s world, data exchange between different systems is a common task. Sometimes you may need to convert data from one format to another. In this article, we will learn how to convert JSON to YAML in Python.
What is JSON?
JSON (JavaScript Object Notation) is a data serialization format designed to be lightweight, easy to read and write, and simple to process for machines. It is based on a subset of the JavaScript programming language. It is widely used in web-based data exchange between a server and a client for sending and receiving data. It is a popular choice for developers who need a lightweight and efficient way to present data due to its simplicity and readability.
JSON data is represented using key-value pairs, enclosed in curly braces {}. Each key-value pair consists of a key (a string) and a value, which can be a string, number, boolean, array, or another object. Arrays in JSON are represented by square brackets [] and can contain multiple values of any data type.
Here’s an example of a JSON object:
{
"book": {
"title": "To Kill a Mockingbird",
"author": "Harper Lee",
"publicationDate": "1960-07-11",
"publisher": {
"name": "J. B. Lippincott & Co.",
"location": "Philadelphia"
},
"ISBN": "978-0-446-31078-0",
"characters": ["Atticus Finch", "Scout Finch", "Jem Finch"],
"genres": ["Southern Gothic", "Bildungsroman"],
"pageCount": 281
}
}
In this example, we have a JSON object that represents information about the book To Kill a Mockingbird by Harper Lee. The object contains a number of key-value pairs associated with the book. These include the title, author, publication date, publisher, ISBN, characters, genres, and pageCount. The publisher key contains another object with details about the book’s publisher. The characters and the genres keys contain arrays of strings representing the book’s characters and genres.
NOTE: This JSON object could be used by a web application to display information about the book, such as on an online bookstore or library catalog.
What is YAML?
YAML stands for Yet Another Markup Language. It is a human-readable data serialization format that is often used for configuration files and data exchange between systems. YAML is often used for configuration files for applications and systems, such as specifying settings for a web application or database configuration. It uses a simple and intuitive syntax, which is based on whitespace indentation to define data structures.
Here’s an example of a YAML format:
book:
title: To Kill a Mockingbird
author: Harper Lee
publicationDate: 1960-07-11
publisher:
name: J. B. Lippincott & Co.
location: Philadelphia
ISBN: 978-0-446-31078-0
characters:
- Atticus Finch
- Scout Finch
- Jem Finch
genres:
- Southern Gothic
- Bildungsroman
pageCount: 281
NOTE: This is the same data that is represented in JSON format.
In this YAML document, we have a book key that contains various sub-keys, each with its values. The publisher key contains another set of sub-keys for the publisher’s name and location. The characters and genres keys contain arrays of values. The YAML document uses indentation to show the nesting of keys and values, making it easier to read and understand than some other data formats like JSON.
Convert JSON to YAML
To convert JSON to YAML in Python, we can make use of two modules:
- json (It is used to load the JSON data into a Python object)
- yaml (It is used to convert the Python object to YAML format)
A step-by-step guide on how to convert JSON to YAML in Python can be found here:
Step 1: Import the required modules
import json
import yaml
As a first step, we import the json and yaml modules that are necessary to convert the JSON data to YAML.
Note: These modules can be installed using the following commands pip install json and pip install pyyaml.
Step 2: Load the JSON data
json_data = '{"book_title": "To Kill a Mockingbird", "author": "Harper Lee", "genres": ["Southern Gothic", "Bildungsroman"]}'
dict_data = json.loads(json_data)
In this step, the JSON data is defined as a string and the json.loads() function is used to load the JSON data into a Python dictionary.
Step 3: Convert the dictionary to YAML
yaml_data = yaml.dump(dict_data)
In this step, the yaml.dump() function is used to convert the Python dictionary to YAML format, which is then stored in the yaml_data variable.
Step 4: Print the YAML data
print(yaml_data)
In this final step, the YAML data is displayed on the console using the print() function.
The complete code to convert JSON to YAML in Python is as follows:
import json
import yaml
json_data = '{"book_title": "To Kill a Mockingbird", "author": "Harper Lee", "genres": ["Southern Gothic", "Bildungsroman"]}'
dict_data = json.loads(json_data)
yaml_data = yaml.dump(dict_data)
print('====================')
print('This is JSON Data')
print('====================\n')
print(json_data)
print('\n\n====================')
print('This is YAML Data')
print('====================')
print(yaml_data)
Output:
====================
This is JSON Data
====================
{"book_title": "To Kill a Mockingbird", "author": "Harper Lee", "genres": ["Southern Gothic", "Bildungsroman"]}
====================
This is YAML Data
====================
author: Harper Lee
book_title: To Kill a Mockingbird
genres:
- Southern Gothic
- Bildungsroman
You may often want to save the JSON data in a YAML file, for this first convert the JSON data to a YAML format. Here’s an example of how to do that in Python:
import json
import yaml
# JSON string to convert
json_data = '{"book_title": "To Kill a Mockingbird", "author": "Harper Lee", "genres": ["Southern Gothic", "Bildungsroman"]}'
# Convert JSON to Python object (dictionary)
dict_data = json.loads(json_data)
print('====================')
print('This is JSON Data')
print('====================')
print(dict_data)
# Convert Python object to YAML
yaml_data = yaml.dump(dict_data, default_flow_style=False)
# Write YAML data to a file
with open('data.yaml', 'w') as yaml_file:
yaml_file.write(yaml_data)
print('\n\n==============================')
print('YAML data is Saved in a file')
print('==============================\n')
Output
====================
This is JSON Data
====================
{'book_title': 'To Kill a Mockingbird', 'author': 'Harper Lee', 'genres': ['Southern Gothic', 'Bildungsroman']}
==============================
YAML data is Saved in a file
==============================
In this example, the yaml.dump() function is used to convert the Python object to YAML format, with default_flow_style set to False to make the output more human-readable. Finally, the YAML data is saved to a file using the write() method. On execution of the above code, you will see a yaml file in your current working directory with the name data.yaml
Convert JSON file to YAML format
Here’s an example of how to convert JSON data from a file to YAML format in Python:
import json
import yaml
# Open the JSON file
with open('data.json') as json_file:
# Load the JSON data
json_data = json.load(json_file)
# Convert JSON to YAML
yaml_data = yaml.dump(json_data, default_flow_style=False)
# Write the YAML data to a file
with open('data.yaml', 'w') as yaml_file:
yaml_file.write(yaml_data)
print('\n\n==============================')
print('YAML data is Saved in a file')
print('==============================\n')
In the above example, first, the JSON file is opened using the open() function and load its contents using json.load(). Then, the yaml.dump() function is used to convert the JSON data to YAML format. After that, the YAML data is saved to a file.
Convert JSON to YAML format Online
There are many online tools available to convert JSON to YAML format. Here are a few popular options:
- JSON to YAML Converter
- Code Beautify JSON to YAML Converter
- Online YAML Tools
To use any of these tools follow these steps:
- Simply paste your JSON data into the input text box or upload your JSON file
- Then click the Convert button.
The tool will then convert your JSON data to YAML format and display it in the output text box. You can then copy the YAML data or download it as a file.
Conclusion
In conclusion, both JSON and YAML are popular data exchange formats that can be used to store and transmit data between different applications and systems. Since JSON is compatible with JavaScript and simple, it is well suited for web-based data exchange, whereas YAML has a human-readable syntax, support for comments, and the ability to represent more complex data structures, and is more commonly used for configuration files, data serialization, and interprocess communication.
The json and yaml modules in Python make it easy to convert JSON data to YAML, so you can use the format which is most suitable for your application. I hope that using the steps outlined in this article, you can easily convert JSON to YAM
Read More:
Easiest Way to Connect Hive with Python:PyHive
How to Modify Python Global Variable inside a Function
Coding Spell | Python Journey | About Us
- How To Find the Length of a List in Python - 16 October 2023
- Python Program to Swap Two Variables - 16 October 2023
- How to Check if a Variable is Null in Python - 14 October 2023