Python try except print error” in Python Programming
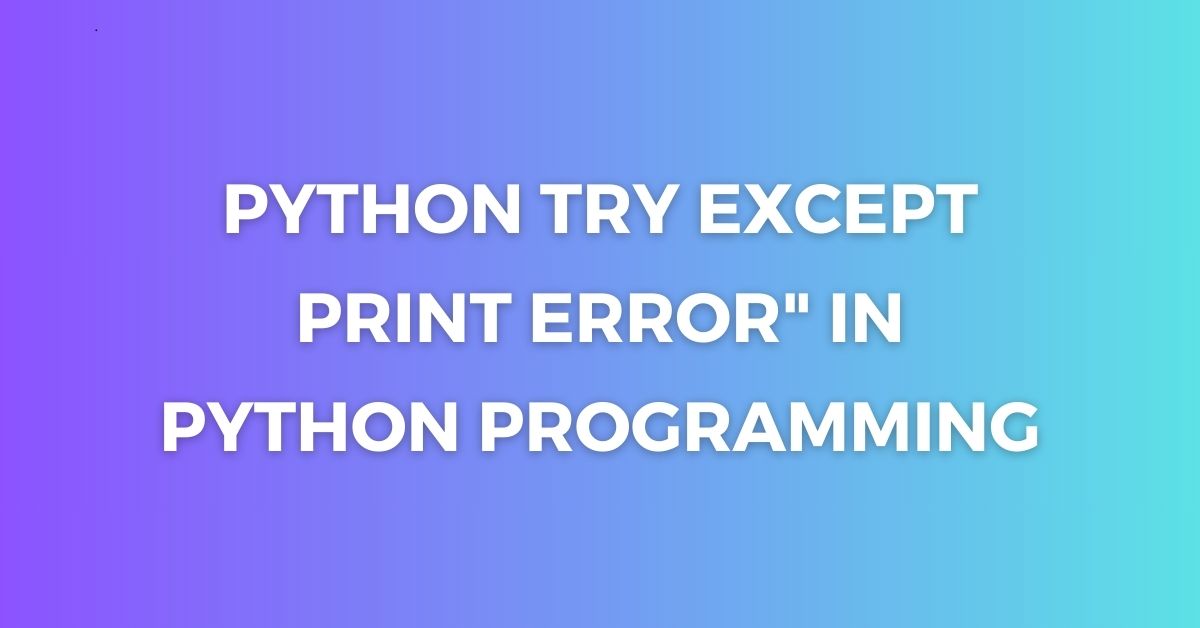
When venturing into the world of Python programming, it’s vital to understand error handling. The “python try except print error” is a crucial concept that can make your programs more robust and user-friendly. Let’s dive deep into this topic!
Prerequisites:
- Basic understanding of Python programming.
- Familiarity with Python functions.
What Will You Learn:
- The concept behind “try” and “except” in Python.
- How to print error messages.
- Practical applications of error handling in Python.
Errors are inevitable in programming. Instead of allowing our program to crash, we can gracefully handle them using the “python try except print error” approach. This method ensures a better user experience and improved code reliability.
Try and Except
What is the “try” block?
The try
block in Python is used to enclose a section of your code that might cause an error. When the code inside the “try” block encounters an error, Python jumps out of the “try” block and looks for the corresponding “except” block.
try:
x = 1 / 0
except:
print("An error occurred!")
In the above code, dividing by zero will cause an error. Instead of crashing, the program will print “An error occurred!”.
The Role of the “except” Block
The “except” block contains the code that should be executed if an error occurs inside the “try” block. It’s like a safety net for your program.
try:
user_input = int(input("Enter a number: "))
except ValueError:
print("That's not a number!")
Here, if the user enters text that’s not a number, the program will print “That’s not a number!” instead of failing.
Printing the Specific Error
In Python, it’s possible to capture and print the specific error message. This can be extremely helpful for debugging purposes or for providing detailed feedback to the user.
try:
y = 1 / 0
except Exception as e:
print(f"Error: {e}")
When this code is run, it will print “Error: division by zero”, informing us about the exact nature of the error.
Practical Applications of Try and Except
Handling File Operations
When working with files, there’s always a chance the file might not exist or might be locked. The “try-except” approach can handle these situations gracefully.
try:
with open("file.txt", "r") as file:
content = file.read()
print(content)
except FileNotFoundError:
print("file.txt not found!")
Network Operations
Network operations are unpredictable. The server might be down, or the connection might drop. Error handling ensures the program doesn’t crash in these scenarios.
import requests
try:
response = requests.get("https://example.com")
print(response.text)
except requests.RequestException:
print("Failed to fetch data from the server!")
FAQs:
- What happens if no “except” block is provided?
If there’s no “except” block and an error occurs inside the “try” block, the program will terminate and display a traceback message. - Can I have multiple “except” blocks?
Yes, you can handle different types of errors by using multiple “except” blocks. - Can I use “try-except” inside functions?
Absolutely! The “try-except” approach can be used anywhere in your Python code, including inside functions. - Is there a way to execute some code whether or not an error occurs?
Yes, you can use the “finally” block, which will be executed no matter what. - How is “try-except” different from using “if-else” for error checking?
While “if-else” checks conditions, “try-except” handles unforeseen errors during code execution, making it more dynamic.
Conclusion:
Mastering the “Python try except print error” approach is a game-changer for budding programmers. It ensures your programs are robust and can handle unexpected situations gracefully. By understanding and implementing this concept, you’re on your way to becoming a proficient Python programmer!
References:
- Python Official Documentation on Errors and Exceptions: https://docs.python.org/3/tutorial/errors.html
Further Reading:
- How To Find the Length of a List in Python - 16 October 2023
- Python Program to Swap Two Variables - 16 October 2023
- How to Check if a Variable is Null in Python - 14 October 2023