TypeError: list indices must be integers or slices not tuple
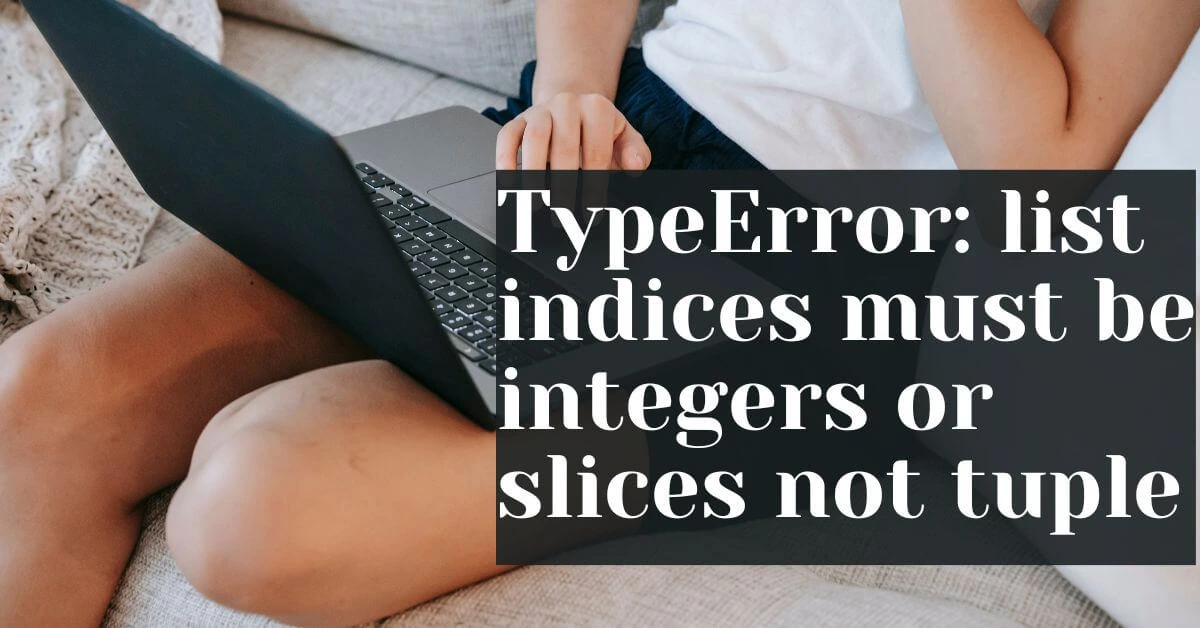
When working with Python lists, you may encounter an error that reads TypeError: list indices must be integers or slices, not tuple. In this article, we will understand this error message and will see different solutions to fix it. Let’s take a closer look.
“TypeError: list indices must be integers or slices, not tuple” is an error that occurs when a list is accessed with a tuple instead of an integer or slice. Lists only permit indexing with integers or slices. To fix the error, the correct index type must be used when accessing the list.
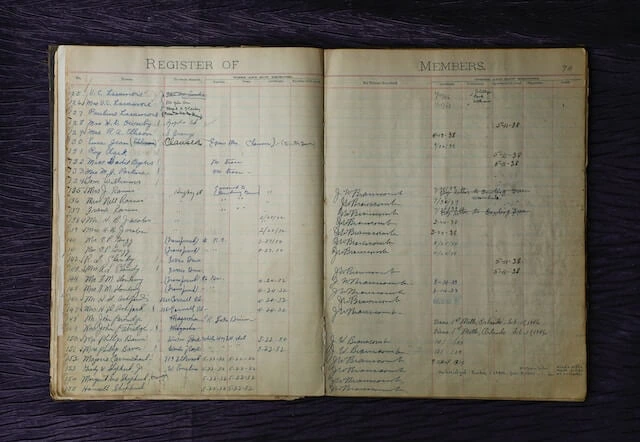
What does the TypeError: list indices must be integers or slices not tuple error mean and how to fix it?
In Python, lists are essentially an ordered collection of items, which can be of any data type. In a list, each element has its own unique index, using which we can manipulate and access the items in the list.
Reason 1: Using a Tuple as an Index for a List
The error message list indices must be integers or slices, not tuple occurs when we try to use a tuple as an index for a list. In Python, a tuple is a collection of ordered and immutable elements. It is similar to lists, but they are immutable, meaning that they cannot be changed once they are created, unlike strings.
Have a look at the below example, to understand this error message better.
my_list = [1, 2, 3, "hi"] my_index = (1, 3) print(my_list[my_index])
Try it yourself -> Online Python editor to run the above example
When you run this code, you will get a TypeError: list indices must be integers or slices, not tuple error. Because, a tuple (1, 3) is used as an index for a list (my_list). Since tuples are not valid indices for lists, Python raises the error.
Solution
To fix this error, unpack the tuple, and use an integer or a slice as the index for the list instead of a tuple.
Unpack the Tuple
my_list = [1, 2, 3, "hi"] my_index = (2, 1) print(my_list[my_index[0]]) print(my_list[my_index[1]])
Output
3
2
In the above code, to access a list element with a tuple, we unpacked the tuple and used its elements as separate indices. Here, my_index[0] is the first element of the tuple, which is 2 which is passed to my_list to access the third element of the list.
Note: List and tuples have a zero-based index which means the first index is at index 0.
Use an Integer
my_list = [1, 2, 3, "hi"] print(my_list[0]) print(my_list[-1])
Output
1
hi
In this example, integer 0 is used as an index to access the first element of the string and -1 is used to access the last element of the list (my_list).
Use Slicing
To extract the range of elements from the list using slicing.
my_list = [1, 2, 3, "hi"]
print(my_list[0:, 2])
Output
TypeError: list indices must be integers or slices, not tuple
In this example, we mistakenly used a comma between the start and the stop indexes [0:, 2], which caused confusion for the Python interpreter and raised the error.
To fix this issue, emit the comma to remove the confusion.
my_list = [1, 2, 3, "hi"]
print(my_list[0: 2])
Output
[1, 2]
We can observe on the execution of the above code error is removed. And the first two elements from the list are returned as [1, 2].
Note: The start index is included and the stop index is not included in the output of slicing.
Reason 2: Improper Separation of List Items
Another reason that can lead to the list indices must be integers or slices, not tuple error is not separating items of a list properly.
my_list = [[1,6], [3,6], [5,7]]
print(my_list[0])
Output
list indices must be integers or slices, not tuple
In this case, the error occurs because the list item [1,6] [3,6] [5,7] are separated with a space that is not correct.
Solution
To fix this error, you should ensure that the items on the list are separated with commas as shown in the below example:
my_list = [[1,6], [3,6], [5,7]] print(my_list[0])
Output
[1,6]
In this code, the list items are separated with commas to avoid errors.
Conclusion
In conclusion, the list indices must be integers or slices, not tuple error can be caused by a variety of factors, including using a tuple instead of an integer or slice to access a list element and improper separation of list items. To fix this error, you should ensure that you are using a valid integer or slice index to access list elements and that the list items are separated properly with commas. Additionally, double-checking your code for any typos or syntax errors can help to prevent this error from occurring in the first place.
Read More:
FIX – TypeError: list indices must be integers or slices not float
How to Send SMS Text Messages Using Python
TypeError: method() takes 1 positional argument but 2 were given
Fix – ImportError: cannot import name force_text from django.utils.encoding
Coding Spell | Python Journey | About Us
- How To Find the Length of a List in Python - 16 October 2023
- Python Program to Swap Two Variables - 16 October 2023
- How to Check if a Variable is Null in Python - 14 October 2023