How to Save Dictionary as JSON Python
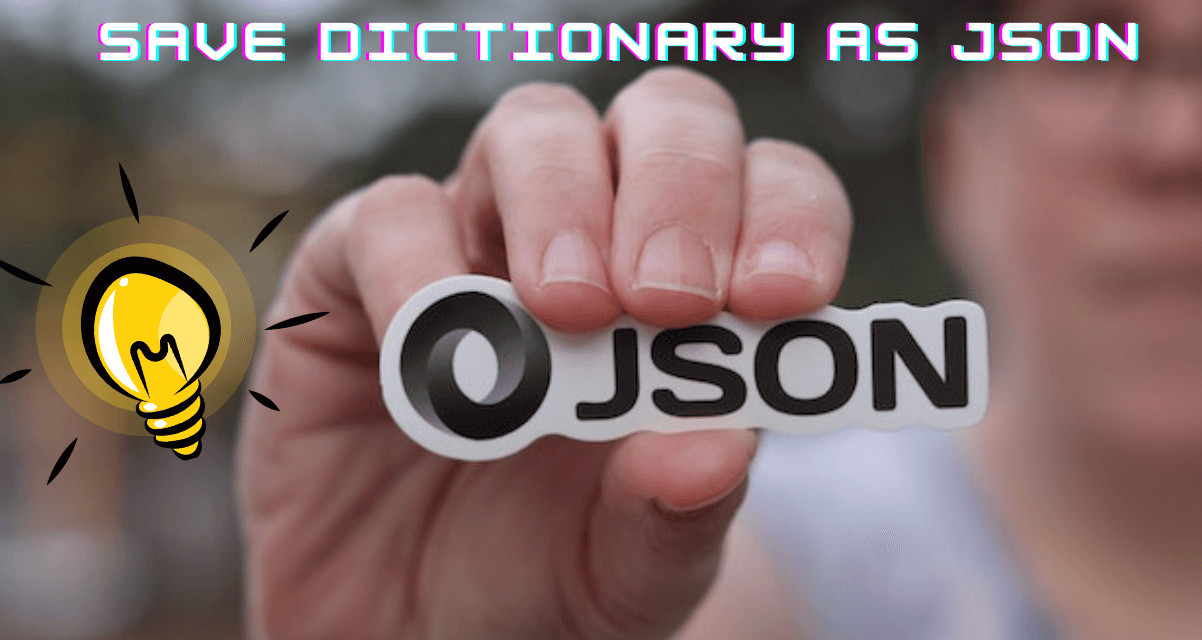
When working with data in Python, dictionaries are a commonly used data structure for storing and manipulating key-value pairs. However, there may be times when you need to convert a dictionary to a JSON (JavaScript Object Notation) format.
The Python JSON module provides a streamlined and effective approach for encoding a dictionary as a JSON formatted string, as well as writing the JSON formatted string to a file via the use of json.dumps() and json.dump() methods. This module proves advantageous in facilitating data exchange between web servers and clients, as well as serving as a reliable means of data storage.
In this tutorial, we will explore how to convert a Python dictionary to a JSON formatted string using the built-in JSON module in Python.
Using json.dumps() Method
To save the dictionary as JSON in Python,
- Use json.dumps() method, available in JSON module. This method serializes a Python object (in this case, a dictionary) to a JSON formatted string and returns it as a string.
Consider the below example to see how to do this:
# Load required Library import json # Create a dictionary Student_dict ={ "roll_number": "01", "student_name": "Burhan", "student_age": "20", "department" : "BSCS" } # save as json json_object = json.dumps(Student_dict, indent = 2) print(json_object)
Output

In the above code snippet, first of all, the JSON library is loaded; after that, a dictionary Student_dict is created which contains information about the student’s roll_number, student_name, student_age and department. Then, json.dumps() method is used to convert a Python dictionary my_dict to a JSON object. Here, the indent parameter is used with value 2, which shows that the JSON object will have 2 spaces for indentation.
Let’s see some more examples to understand:
# Load required Library import json # Create a dictionary my_dict ={ "name": "Ayesha", "age": "24", "email" : "ayesha@outlook.com", "city": "Malmo", "country" : "Sweden", } # save as json json_object = json.dumps(my_dict, sort_keys=True) print(json_object)
Output

In this code, dictionary my_dict is created, which is converted to JSON formatted string using json.dumps() method. We can observe here optional parameter sort_keys is set to True, which is used to sort the keys of JSON objects in alphabetical order.
Now let’s consider a scenario where we need to convert a nested dictionary to JSON format. Below example will show how to do this.
import json # Define a nested Python dictionary my_dict = { "person": { "name": "Haram", "age": 17, "address": { "street": "456 Main St", "city": "New York", "state": "NY", "zip": "34434" } } } # Convert the dictionary to a JSON string json_str = json.dumps(my_dict, indent=2) print(json_str)
Output

In the above example, the dictionary my_dict contained nested dictionary addresses. Here, JSON.The dumps () method converts the dictionary to JSON formatted string. In the result, we can observe nested dictionary address converted to JSON object enclosed within curly braces inside the outer JSON object.
Using json.dump() Method
To save the dictionary as JSON in Python,
- Use json.dump() method available in JSON module. This method serializes a Python object (in this case, a dictionary) to a JSON formatted string and writes it to a file-like object fp
Check the below example to see how to use json.dump() method to convert the dictionary to JSON.
import json # Create a dictionary my_dict ={ "name": "Ayesha", "age": "24", "email" : "ayesha@outlook.com", "city": "Malmo", "country" : "Sweden", } # Open a file for writing with open("my_dict.json", "w") as fp: # Write dictionary to file as JSON json.dump(my_dict, fp) print("Dictionary saved as JSON file.")
Output

In the code above, we imported the JSON module and defined a dictionary called my_dict. Next, a file called my_dict.json is opened in write mode using a with the statement. Inside the with block, the json.dump() function is used to write the dictionary to the file in JSON format. The first argument to json.dump() is the dictionary we want to save, and the second argument is the file object we want to save it to. Finally, a message Dictionary is saved as a JSON file. is printed to the console to indicate that the process is complete.
After running the code, you should see a new file called my_dict.json in your current working directory. The file’s contents should be a JSON-encoded version of the my_dict dictionary.
Note: The json.dumps() method can also be used to convert a python dictionary to JSON string and then write the string to a file.
# Load required Libarary import json # Create a dictionary Student_dict ={ "roll_number": "01", "student_name": "Burhan", "student_age": "20", "department" : "BSCS" } # save as json json_object = json.dumps(Student_dict, indent = 2) # write the JSON string to a file with open('Student_dict.json', 'w') as f: f.write(json_object) print("Dictionary saved as JSON file.")
Output

This code demonstrates how to convert a Python dictionary to a JSON formatted string, and then write it to a file using the JSON module. The json.dumps() method is used to convert the Student_dict dictionary to a JSON formatted string. The first argument to json.dumps() is the dictionary we want to convert, and the second argument indent=2 is an optional argument that specifies the number of spaces to use for indentation in the resulting JSON string as we discussed above. This makes the output easier to read for humans.
The resulting JSON formatted string is then assigned to a variable called json_object.
Finally, the json_object string is written to a file called Student_dict.json using a with the statement and the write() function. The with statement is used to ensure that the file is closed properly after the writing is complete.
After running this code, a file called Student_dict.json will be created in the current working directory, containing the JSON representation of Student_dict with proper indentation.
Also, Check here to learn how to return SQL data in JSON format python
Conclusion:
This article covered how to save a dictionary as JSON Python. The Python JSON module offers a simple and efficient way to save a dictionary as a JSON formatted string or to write a JSON formatted string to a file.
This is especially beneficial for data exchange between web servers and clients and data storage. You can easily convert your Python dictionaries to JSON format and save them as files by following the simple steps outlined in this tutorial. The JSON module is valuable due to its ease of use and flexibility.
Good Luck with your Learning !!
Related Topics:
Difference between fetchone and fetchall in Python
Resolve “ImportError: Attempted Relative Import with No Known Parent Package”
How to Modify Python Global Variable inside a Function
- How to Fix – TypeError: only size-1 arrays can be converted to Python scalars - 16 October 2023
- How to Implement d’wave qbsolv in Python - 16 October 2023
- Resolve Javascript error: ipython is not defined - 15 October 2023