Resolve “ImportError: Attempted Relative Import with No Known Parent Package”
As a Python programmer, You might have some idea about packages and modules. In this article, We will understand the error “ImportError: attempted relative import with no known parent package
” and the efficient way to resolve it
To fix “ImportError: Attempted Relative Import with No Known Parent Package,” ensure proper package structure, include __init__.py
files, and run as a package or use absolute imports.
What is ‘ImportError: Attempted Relative Import with No Known Parent Package’?
ImportError: attempted relative import with no known parent package
error message means the Python interpreter tries to use a relative import statement but the interpreter cannot determine the parent package.
Relative imports are used to import modules or packages that are in the same package or subpackage as the current module. This helps us to organize code within a package structure and give us a better way to reference other modules or packages within the same package.
Before proceeding further, Let’s understand, What is Relative and Absolute Import in python
Absolute Imports
Let’s consider, our Python package structure below, So that it would be helpful for you to compare the examples
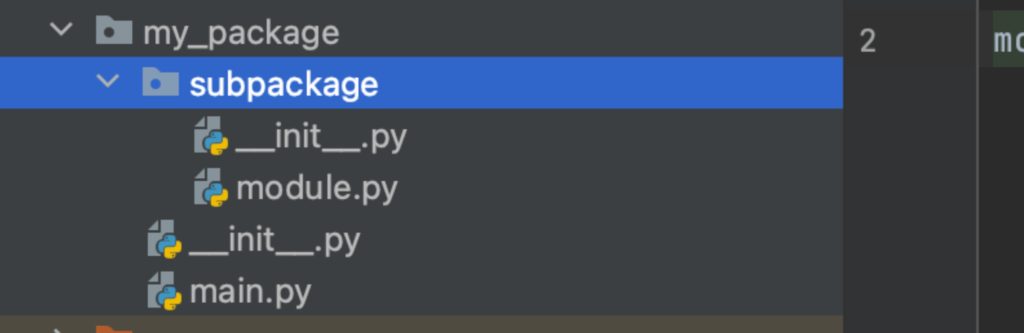
In absolute import, We refer modules or packages using the full path from the project’s root directory. It is basically to provide a complete location of the module or package that you want to import. It is mostly used when you are trying to import packages from external libraries or from different projects
Example:
import my_package.subpackage.module
Let’s compare the screenshot with the example, my_package.subpackage.module
is the full path to the module you wanted to import. Basically, it is a straight forward method by providing the compare path of the package or modules
Relative Imports
Let’s use the same example screenshot shared above, to understand Relative Imports in Python
Relative import, Will refers to importing modules or packages based on their location relative to the current module. It is denoted by a dot(.) followed by a module or package name. The number of dots represents the level of the parent package.
Single (.) for the current directory and (..) is one step higher in the package hierarchy
Example:
from .subpackage import module
dot (.) signifies that the import is relative to the current package. The subpackage is a subpackage within the same package as the current module and module
is the module you want to import from that subpackage.
How to Replicate the Issue
To replicate the ImportError: attempted relative import with no known parent package
error, follow these steps:
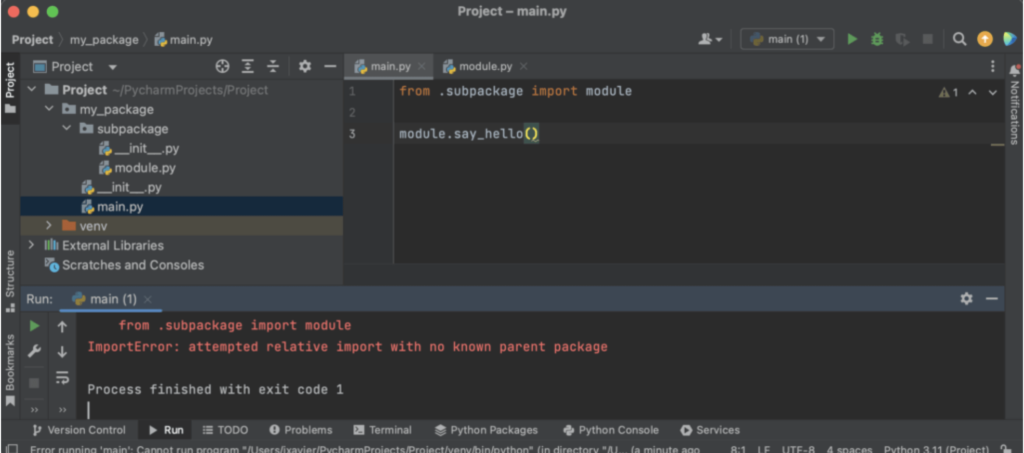
For this example, I am using pycharm IDE, you can use the same steps for running the Python in the terminal as well
- Create a new directory called
my_package
on your pycharm IDE. - Inside the
my_package
directory, create two files:main.py
andsubpackage
- Open
main.py
and add the following code:
from .subpackage import module
module.say_hello()
- Inside the
directory, create a filesubpackage
module.py
- Open
and add the below code:module.py
def say_hello():
print("Hello from subpackage")
- Navigate to the my_package -> main.py -> Run (From Pycharm IDE) – This will replicate the issue
ImportError: attempted relative import with no known parent package
How to Resolve the Issue
To resolve the ImportError: attempted relative import with no known parent package
error, We can try the below methods
Using setup.py
Create a setup.py file and add all the packages that you need to keep it as global packages, Which means, you can access any packages from any other packages
Create setup.py
from setuptools import setup, find_packages setup(name = 'package_two', packages = find_packages())
As I am using pycharm IDE to run these examples, To create a setup.py file will be a little different
Use this link to find the step-by-step guide to creating setup.py files
Once have installed the setup.py, Your package will be available globally So that you can directly import as below
from subpackage import module
module.say_hello()
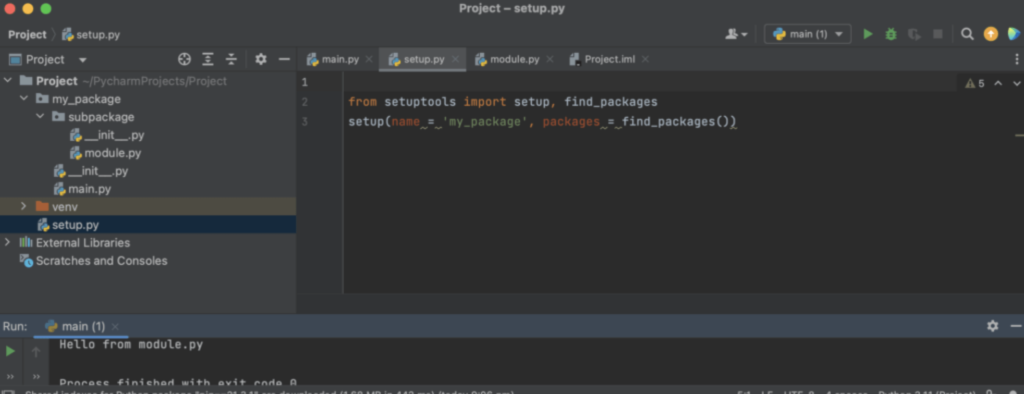
Verify the Package Structure
Make sure that your package structure is set up correctly. Each package directory should contain a __init__.py
file, which signifies that the directory is a Python package. Verify that the package structure looks like this:
my_package/
__init__.py
main.py
subpackage/
__init__.py
module.py
If you are not seeing the __init__.py
file, You can directly create a empty file under the directories with exact same name
Check Import Statements
If you are using relative imports ensure the syntax is valid and the (.) specified properly. Also keep in mind, relative imports should only be used within packages. If you try to use them outside of a package or when running a script as a standalone module, you may encounter the “ImportError: attempted relative import with no known parent package” error.
from .subpackage import module
Execute the Script from the Correct Directory
Make sure you are running the script from the appropriate directory. Relative imports depend on the current working directory. Navigate to the parent directory of my_package
and execute the script below

If you are using the terminal to execute the program, Make sure to navigate to the parent package and run the Python code
Use Absolute Imports
If you are attempting to run a script as a standalone module (not as part of a package), relative imports will not work. In such cases, consider one of the following options:
- Restructure Your Code: Modify your code to form a proper package structure. Create a
__init__.py
file in the directory containing your script and organize your code into appropriate subdirectories. - Use Absolute Imports: Instead of using relative import statements, specify the full package path when importing modules or packages. For example:
from my_package.subpackage import module
By using absolute imports, you avoid the need for relative import statements altogether.

Conclusion
The ImportError: attempted relative import with no known parent package
error occurs when you use a relative import statement without a well-defined parent package structure. By ensuring a proper package structure, using correct import statements, and executing the script from the correct directory, you can resolve this error and effectively utilize relative imports within your Python projects.
Good Luck with your Learning !!
Related Topics:
Fix – “ImportError: No module named pip” in Python
Python List Comprehension IF: A Comprehensive Guide
Could not import azure.core Error in Python
How to Find N Root of a Number – Python
- How to Fix – TypeError: only size-1 arrays can be converted to Python scalars - 16 October 2023
- How to Implement d’wave qbsolv in Python - 16 October 2023
- Resolve Javascript error: ipython is not defined - 15 October 2023