AttributeError: ‘dict’ object has no attribute ‘read’

When working with Python, you might encounter an AttributeError with the message “dict’ object has no attribute ‘read'”. This error can be quite confusing, especially for those who are new to the language. In this article, we’ll explore what this error means, its common causes, and how to fix it.
The “AttributeError: ‘dict’ object has no attribute ‘read'” occurs when trying to access a non-existent attribute. To fix, ensure you’re working with the correct object type and attribute. Alternatively, create a custom class that behaves like a file object and use it to read from the dictionary.
Let’s first discuss about dictionary in Python and its use
What is a Dictionary in Python?
In Python, a dictionary is an unordered collection of key-value pairs, where each key is unique. Dictionaries are used in place of lists when working with large amounts of data. It stores the data as a {key: value} structure and it is denoted as {}
dictionary={"name":"Share"}
Check out the article “Learn Python Dictionary and How to Create a Nested dictionary?” to know more about dictionary
Why does the ‘AttributeError: ‘dict’ object has no attribute ‘read” occur?
Let’s start by breaking down the error message. In Python, everything is an object, and objects have attributes. An attribute is simply a value associated with an object that can be accessed using dot notation. For example, a list object in Python has attributes such as “append”, “extend”, “insert”, and so on. When you try to access an attribute that doesn’t exist for an object, you’ll get an AttributeError.
The error message “dict’ object has no attribute ‘read'” indicates that you’re trying to access the “read” attribute on a dictionary object, but dictionaries don’t have a “read” attribute. This error typically occurs when you’re trying to treat a dictionary like a file object, which can be read from or written to using methods like “read”, “readline”, “write”, and so on.
Example 1
pythonCopy codemy_dict = {'key1': 'value1', 'key2': 'value2'}
with open('file.txt', 'r') as f:
my_dict = f.read()
Output:
>>> my_dict = {'key1': 'value1', 'key2': 'value2'} >>> with open('file.txt', 'r') as f: ... my_dict = f.read() ... >>> print(my_dict) This is some example text in a file. It can be anything you want it to be. You can use this file to test reading text from a file.
In the above example, we’re trying to read the contents of a file and store them in a dictionary. However, we’re assigning the result of f.read() directly to the my_dict variable, which overwrites the original dictionary. As a result, my_dict is no longer a dictionary, but a string containing the contents of the file. If we then try to access an attribute like “read” on my_dict, we’ll get the “dict’ object has no attribute ‘read'” error.
Example 2
my_dict = {'key1': 'value1', 'key2': 'value2'} my_dict.read()
Output:
AttributeError: 'dict' object has no attribute 'read' Process finished with exit code 1
In this example, we’re simply trying to access the “read” attribute on a dictionary object. However, as we mentioned earlier, dictionaries don’t have a “read” attribute, so we’ll get the “dict’ object has no attribute ‘read'” error.
Reference:
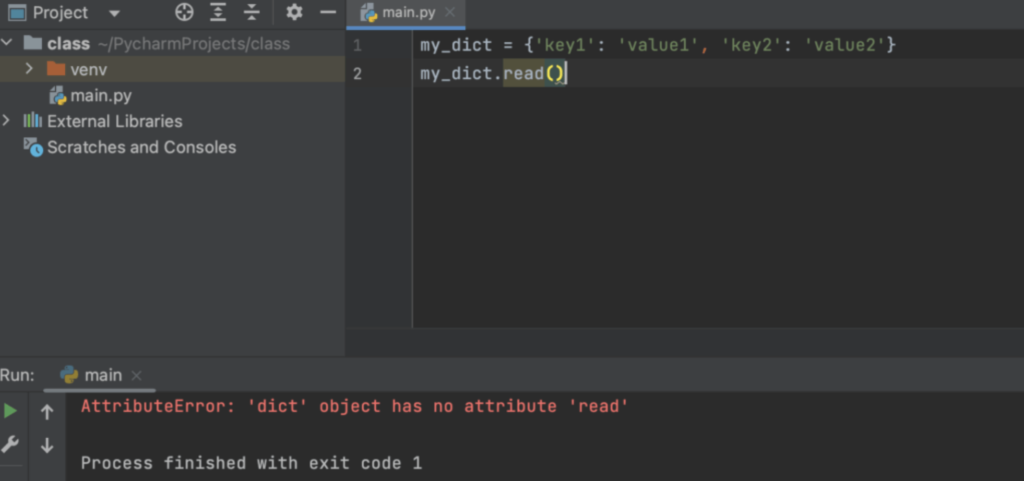
How to Fix the Error
Using file object
To fix the “dict‘ object has no attribute ‘read‘” error, you need to make sure you’re working with the correct type of object. If you’re trying to read from a file, for example, you should use a file object rather than a dictionary.
Example 1:
my_dict = {'key1': 'value1', 'key2': 'value2'} with open('file.txt', 'r') as f: file_contents = f.read() my_dict['file_contents'] = file_contents
Output:
% python3 Python 3.11.0 (v3.11.0:deaf509e8f, Oct 24 2022, 14:43:23) [Clang 13.0.0 (clang-1300.0.29.30)] on darwin Type "help", "copyright", "credits" or "license" for more information. >>> my_dict = {'key1': 'value1', 'key2': 'value2'} >>> with open('file.txt', 'r') as f: ... file_contents = f.read() >>> my_dict['file_contents'] = file_contents >>> print(my_dict) {'key1': 'value1', 'key2': 'value2', 'file_contents': 'This is some example text in a file.\nIt can be anything you want it to be.\nYou can use this file to test reading text from a file.\n'} >>>
In this version, we’re creating a new variable called file_contents to store the contents of the file, rather than overwriting the my_dict variable. We then add the contents of the file to the dictionary using a new key called “file_contents“.
If you’re still encountering the error after ensuring that you’re working with the correct type of object, make sure that the object you’re working with actually has the attribute you’re trying to access. You can use the “dir” function to list all the attributes of an object:
my_dict = {'key1': 'value1', 'key2': 'value2'} print(dir(my_dict))
Output:
['__class__', '__class_getitem__', '__contains__', '__delattr__', '__delitem__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__getitem__', '__getstate__', '__gt__', '__hash__', '__init__', '__init_subclass__', '__ior__', '__iter__', '__le__', '__len__', '__lt__', '__ne__', '__new__', '__or__', '__reduce__', '__reduce_ex__', '__repr__', '__reversed__', '__ror__', '__setattr__', '__setitem__', '__sizeof__', '__str__', '__subclasshook__', 'clear', 'copy', 'fromkeys', 'get', 'items', 'keys', 'pop', 'popitem', 'setdefault', 'update', 'values'] Process finished with exit code 0
Above are the available list all the attributes available for dictionary
Using “json” module
To fix the error is to use a different method for reading from or writing to a file. For example, you can use the “json” module to read and write JSON files:
Example:
import json my_dict = {'key1': 'value1', 'key2': 'value2'} with open('file.json', 'r') as f: file_contents = json.load(f) my_dict['file_contents'] = file_contents
Output:
>>> import json >>> >>> my_dict = {'key1': 'value1', 'key2': 'value2'} >>> with open('file.json', 'r') as f: ... file_contents = json.load(f) >>> my_dict['file_contents'] = file_contents >>> print(my_dict) {'key1': 'value1', 'key2': 'value2', 'file_contents': 'This is some example text in a file.\nIt can be anything you want it to be.\nYou can use this file to test reading text from a file.\n'} >>>
In this example, we’re reading from a JSON file instead of a text file, and we’re using the json.load() method to load the contents of the file into a dictionary.
Conclusion
The “dict’ object has no attribute ‘read’” error can be confusing, but it’s simply a result of trying to access an attribute that doesn’t exist for an object. To fix the error, make sure you’re working with the correct type of object and that the object actually has the attribute you’re trying to access. Additionally, consider using different methods for reading from or writing to files if you’re encountering this error while working with files.
Good Luck with your Learning !!
Related Article:
AttributeError: ‘dict’ object has no attribute ‘append’
How to Calculate SHA256 Hash of a File in Python
AES 256 Encryption and Decryption in Python
- How to Fix – TypeError: only size-1 arrays can be converted to Python scalars - 16 October 2023
- How to Implement d’wave qbsolv in Python - 16 October 2023
- Resolve Javascript error: ipython is not defined - 15 October 2023