How to Find N Root of a Number – Python
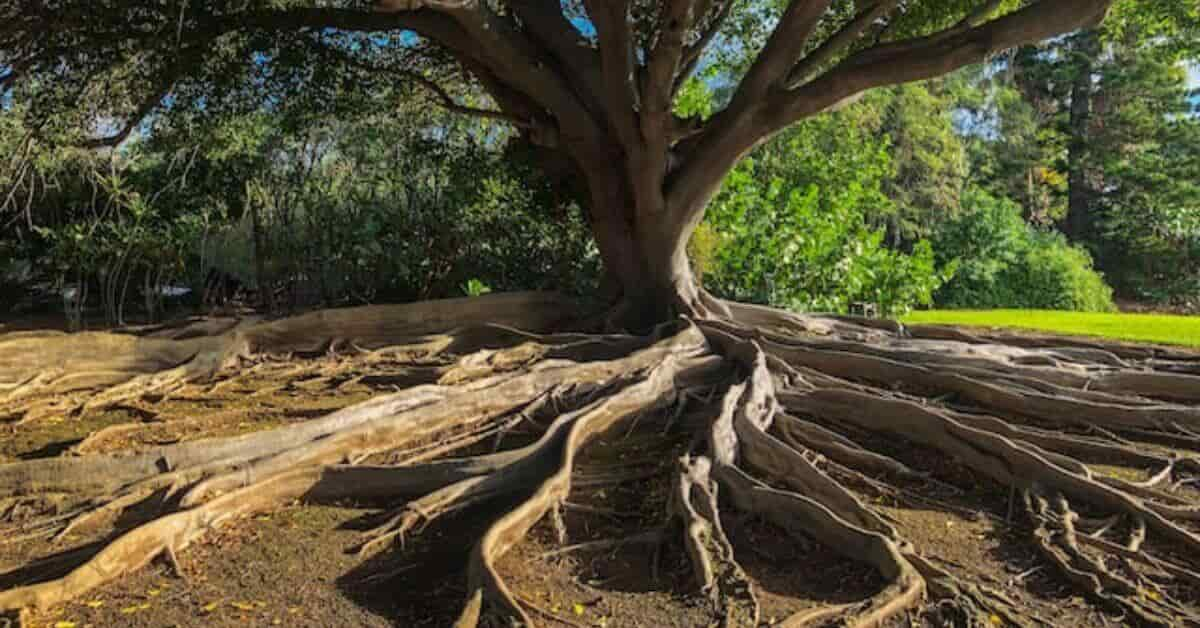
Let’s start with the basics before showcasing different Python approaches – How to find the N root of a number, each accompanied by clear explanations and examples.
Understanding the Nth Root of a Number
In mathematics, the nth root of a number refers to a value that, when raised to the power of n, results in the original number. It is a concept that extends beyond the familiar territory of square and cube roots, inviting us to explore higher roots. Let’s break it down with examples:
- Square root (n=2): The square root of 64 is 8, as 8² (8 multiplied by itself) equals 64.
- Cube root (n=3): The cube root of 8 is 2, because 2³ (2 multiplied by itself twice) gives us 8.
- Fourth root, fifth root, and beyond: This principle can be extended to higher roots, diving deeper into the exciting world of mathematics.
Methods to Calculate the Nth Root in Python
Python offers several approaches to find the nth root of a number. We will explore three popular methods, ensuring to break down each step for a full understanding:
1. Using the Exponentiation Operator
Python’s exponentiation operator (**
) serves as our first tool. To find the nth root, we raise the number to the power of 1/n
. Below, find a script that calculates the cube root of 8:
num = 8
n = 3
result = num ** (1/n)
print(result)
# Output: 2.0
In this script:
- We define the number (
num
) and the root (n
) we are interested in. - We calculate the nth root using the formula
num ** (1/n)
. - We print the result, obtaining 2.0, confirming that the cube root of 8 is 2.
2. Leveraging the math Library
The math
library in Python houses the .pow()
function, a potent tool in our endeavor. It requires two parameters: the base number and the inverse of the root (1/n). Let’s demonstrate this by finding the 100th root of 10:
import math
num = 10
n = 100
result = math.pow(num, 1/n)
print(result)
# Output: 1.023292992280754
Explanation:
- We import the
math
library to access the.pow()
function. - We find the 100th root of 10 using the formula
math.pow(num, 1/n)
.
3. Utilizing the numpy Library
Our third method involves the numpy
library, utilizing its .power()
function which operates similarly to math.pow()
but offers additional functionalities, such as handling array-based calculations. Let’s see it in action with an array of numbers:
import numpy as np
arr = np.array([8, 64, 125])
n = 3
result = np.power(arr, 1/n)
print(result)
# Output: [2. 4. 5.]
In this script:
- We use
numpy
to work with an array of numbers. - We find the cube root of each number in the array, demonstrating
numpy
’s efficiency with arrays.
Conclusion
In this guide, we have navigated the mathematical concept of nth roots, laying a solid foundation with clear definitions and progressively building up to Python scripts that find nth roots using various methods. We hope this serves as a useful tool in your Python programming journey, offering value to both beginners and experienced coders alike.
Feel free to experiment with the scripts, altering the values to find different roots and enhance your understanding further. Happy coding!
FAQs
Q1: What is the Nth root of a number?
A1: The Nth root of a number is a value that, when raised to the power of N, equals the original number. For example, the square root of 9 is 3, because 3² = 9; similarly, the cube root of 27 is 3, because 3³ = 27.
Q2: How can I calculate the Nth root of a number in Python?
A2: You can calculate the Nth root of a number in Python using the **
operator or the pow()
function. Here’s how you can do it:
def nth_root(num, n):
return num ** (1/n)
# Example usage
root = nth_root(27, 3)
print(root) # Output: 3.0
Q3: What libraries in Python can assist in finding the Nth root?
A3: While Python’s built-in math library doesn’t offer a specific function for finding Nth roots, it does offer other functions like pow()
and sqrt()
which can be useful. Moreover, you can use NumPy, a powerful library for numerical computations, which offers various mathematical functions including finding Nth roots using numpy.nth_root()
.
Q4: How do I find the Nth root of complex numbers in Python?
A4: To find the Nth root of complex numbers in Python, you can use the cmath
library which is designed to handle complex numbers. Here is an example:
import cmath
def nth_root(num, n):
return cmath.pow(num, 1/n)
# Example usage
root = nth_root(27 + 8j, 3)
print(root) # Output: (2.2795070569547775+1.0978562826823509j)
Q5: Can I find the Nth root of negative numbers in Python?
A5: Yes, you can find the Nth root of negative numbers in Python by using complex numbers. Python’s cmath
library will allow you to work with negative numbers when finding roots, as shown in the answer to Q4 above.
Q6: How can I handle errors and exceptions when finding the Nth root in Python?
A6: When writing a function to find the Nth root in Python, you may want to include error handling to manage inputs that could produce errors, such as negative numbers or zero. Here’s how you can handle errors gracefully:
def nth_root(num, n):
try:
return num ** (1/n)
except ZeroDivisionError:
return "N cannot be zero"
except ValueError as e:
return str(e)
# Example usage
root = nth_root(-27, 3)
print(root) # Output: a message indicating a math domain error
Q7: How do I find the Nth roots of a polynomial in Python?
A7: To find the Nth roots of a polynomial in Python, you can use numerical methods libraries like NumPy or SciPy. These libraries offer functions to find roots of polynomials. For instance, numpy.roots()
can be used to find all roots of a polynomial:
import numpy as np
# Define the coefficients of the polynomial
coefficients = [1, 0, -9]
# Find the roots of the polynomial
roots = np.roots(coefficients)
print(roots) # Output: array of polynomial roots
Additional Notes
Make sure to explore Python’s math
, cmath
, and numpy
libraries for a range of functions that can assist you in various mathematical computations, including finding the Nth root of numbers.
Read More:
1: ssl module in Python is not available
- How to Fix – TypeError: only size-1 arrays can be converted to Python scalars - 16 October 2023
- How to Implement d’wave qbsolv in Python - 16 October 2023
- Resolve Javascript error: ipython is not defined - 15 October 2023