Python Hangman Code – Building a Classic Game from Scratch
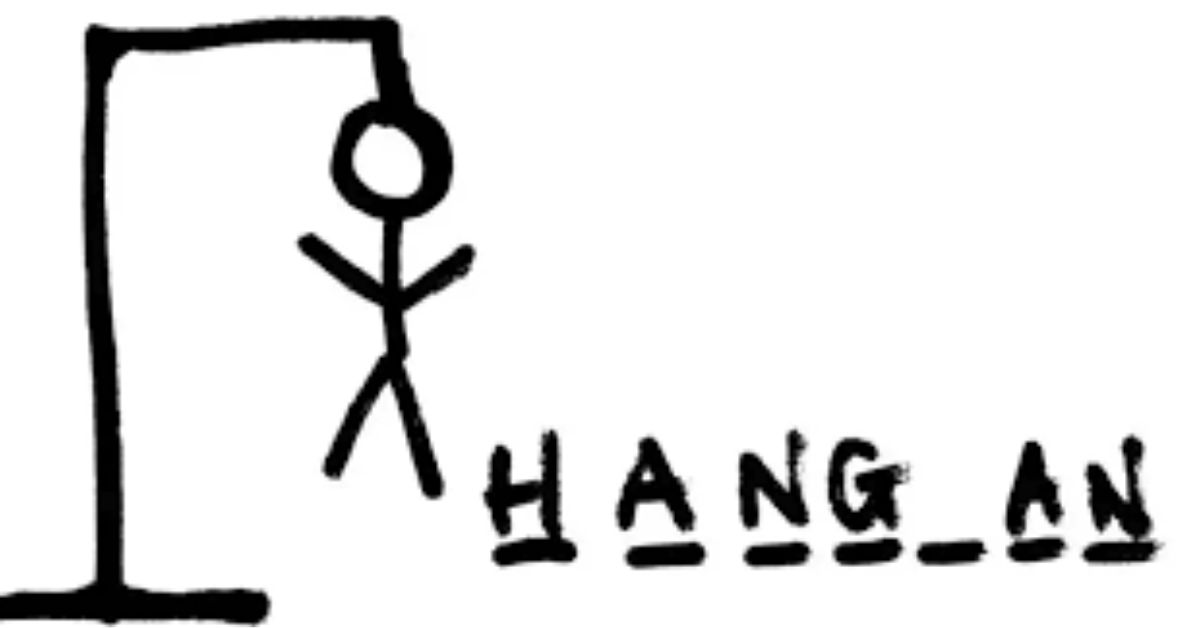
The Python Hangman code is a fantastic project to embark on, offering an engaging way to deepen your understanding of Python while having fun. In this article, we guide you through creating your very own Hangman game using Python, ensuring a detailed yet simple, understandable, Let’s dive in!
Understanding the Basics of Hangman
Hangman is a classic word-guessing game that involves guessing letters one by one to reveal a hidden word before the hangman is fully drawn. Python, with its readability and simplicity, is the perfect language for building this game. We will leverage Python’s fundamental concepts like loops, conditionals, and strings to create our Python Hangman code.
Setting up Your Development Environment
Before we get started, ensure you have a Python environment set up. You can download the latest version from the Python official website. Additionally, a text editor like Visual Studio Code or PyCharm will be beneficial.
Developing the Python Hangman Code
To build our Python Hangman code, we will proceed with the following steps:
Step 1: Import Necessary Modules
import random
We use the random
module to select a random word from our list of words.
Step 2: List of Words
words = ["PYTHON", "HANGMAN", "CODING", "DEVELOPER"]
Create a list of words that will be used in the game. Feel free to add as many words as you want.
Step 3: Initialize Variables
word = random.choice(words)
guessed_letters = []
attempts = 6
We choose a random word from the list and initialize the number of attempts and an empty list to store the guessed letters.
Step 4: The Game Loop
while attempts > 0:
incomplete_word = "".join([letter if letter in guessed_letters else "_" for letter in word])
print(incomplete_word)
print(f"You have {attempts} attempts remaining.")
guess = input("Please enter a letter: ").upper()
if guess in word:
guessed_letters.append(guess)
if all(letter in guessed_letters for letter in word):
print(f"Congratulations! You've guessed the word: {word}")
break
else:
attempts -= 1
else:
print(f"Sorry, you've run out of attempts. The word was: {word}")
In this loop:
- We create an incomplete word with the guessed letters revealed and unknown letters as underscores.
- We allow the player to input their guesses, decreasing the attempts for wrong guesses.
- We check for a win condition by verifying if all the letters of the word are guessed.
Detailed Explanation
In our Python Hangman code:
random.choice(words)
is used to randomly select a word from our list.- The
incomplete_word
variable displays the current state of the word being guessed. - We’ve incorporated a loop to continually prompt the user for inputs until the attempts run out or the word is guessed.
Conclusion
You’ve successfully navigated through the creation of a Python Hangman code, a fascinating endeavor for coders ranging from beginners to the advanced. It’s not just a triumph of building a classic game from scratch, but a leap in understanding Python more intimately. Remember, the beauty of coding lies in the endless possibilities; feel free to add more features such as score tracking, levels of difficulty, or a larger word bank to enhance your game.
Embrace the Python Hangman code project as a fantastic learning pathway and a demonstration of Python’s flexibility and user-friendliness. Now, with your freshly minted game, it’s time to challenge your friends to a game of Hangman, showcasing your Python prowess. Happy coding!
FAQs
1. Can I add more words to the hangman game?
Yes, you can definitely add more words to your hangman game. In the word_list
variable, you can include as many words as you’d like. Just ensure each word is encapsulated by quotation marks and separated by a comma. For instance:
word_list = ["PYTHON", "HANGMAN", "CODING", "PYTHONIC", "DEVELOPER", "JAVASCRIPT", "DATABASE"]
2. How can I change the number of attempts allowed in the game?
Adjusting the number of attempts in the game is straightforward. You’ll find a variable named attempts
in the script, which is initially set to 6. You can change this number to any positive integer to increase or decrease the difficulty level. For example:
attempts = 8
3. Can I personalize the messages displayed during the game?
Yes, you can personalize all the messages displayed during the game by modifying the print
statements in the Python script. For instance, to change the congratulatory message, find the following line and alter the message within the quotation marks:
print(f"Congratulations! You guessed the word: {word_to_guess}")
4. How do I ensure that the game accepts only single-letter inputs?
The game already contains a validation check to ensure that players enter only single letters. This is done using the following condition:
if guess in guessed_letters or not guess.isalpha() or len(guess) != 1:
print("Invalid guess. Try again.")
continue
In this snippet, not guess.isalpha()
checks that the input is a letter, and len(guess) != 1
verifies that it’s a single character.
5. Can I run this game in a web browser or make a graphical version?
Currently, the hangman game is designed to run in a Python environment, like a Python file executed through a command line or an integrated development environment (IDE).
To make it run in a web browser or create a graphical version, you would need to integrate it with a web framework like Flask or Django for a web version, or a graphical user interface (GUI) library like Tkinter or PyQt for a desktop application. These integrations are more advanced and require knowledge in web development or GUI development in Python.
6: What are the rules of the Hangman game?
In the Hangman game, one player (or the computer) selects a word, and the other player tries to guess the word one letter at a time. Each incorrect guess results in a part of the ‘hangman’ figure being drawn. The game ends when the player guesses the word correctly or when the hangman figure is completely drawn, which occurs after a predetermined number of incorrect guesses.
7: How do I start the Python Hangman game?
To start the Python Hangman game, you would need to have Python installed on your system. Once installed, download or clone the Python Hangman code repository, navigate to the directory where the hangman.py
file is located using a terminal or command prompt, and run the command python hangman.py
to start the game.
8: Can I add new words to the Hangman game’s word list?
A4: Yes, you can add new words to the Hangman game’s word list. Open the Python file that contains the word list (usually a list or array within the script) and add your new words, following the existing format.
9: How can I customize the number of allowed incorrect guesses?
A5: To customize the number of allowed incorrect guesses, locate the variable in the code that sets this number (usually named something like max_attempts
or allowed_guesses
) and change its value to your preferred number of guesses.
10: Can I play the Hangman game with a friend remotely?
A6: The basic Python Hangman code usually supports single-player gameplay with the computer. To play with a friend remotely, you would need to modify the code to support multiplayer gameplay over a network, which could be a significantly more complex task involving networking knowledge and additional Python packages.
11: How do I fix the error “Python is not recognized as an internal or external command”?
This error occurs because Python is not installed on your system or the Python installation path is not added to the system’s PATH environment variable. To fix this, first ensure Python is installed on your system by downloading it from the official website. During installation, check the box that says “Add Python to PATH” to automatically add Python to your system’s PATH environment variable.
12: Can I input more than one letter at a time while playing?
Typically, the Hangman game is designed to accept one letter at a time. However, some versions of the game might allow you to guess the entire word in one go. You would need to check the specific instructions for the Python Hangman code you are using to know if this feature is supported.
13: Can I play the Python Hangman game on any operating system?
As long as you have a Python interpreter installed, you should be able to play the Python Hangman game on any operating system that supports Python, including Windows, macOS, and Linux.
14: Is there any way to get hints during the game?
Whether or not you can get hints during the game depends on the specific implementation of the Python Hangman code. Some versions may offer a hint feature, where you can get a hint after a certain number of incorrect guesses. Check the game’s instructions or help menu to see if this feature is available.
Read More:
1: Python Create Directory If It Doesn’t Exist
2: Fix Python Error: Legacy-Install-Failure
3: Random Word Generator Python
- How to Fix – TypeError: only size-1 arrays can be converted to Python scalars - 16 October 2023
- How to Implement d’wave qbsolv in Python - 16 October 2023
- Resolve Javascript error: ipython is not defined - 15 October 2023