List of Lists in Python
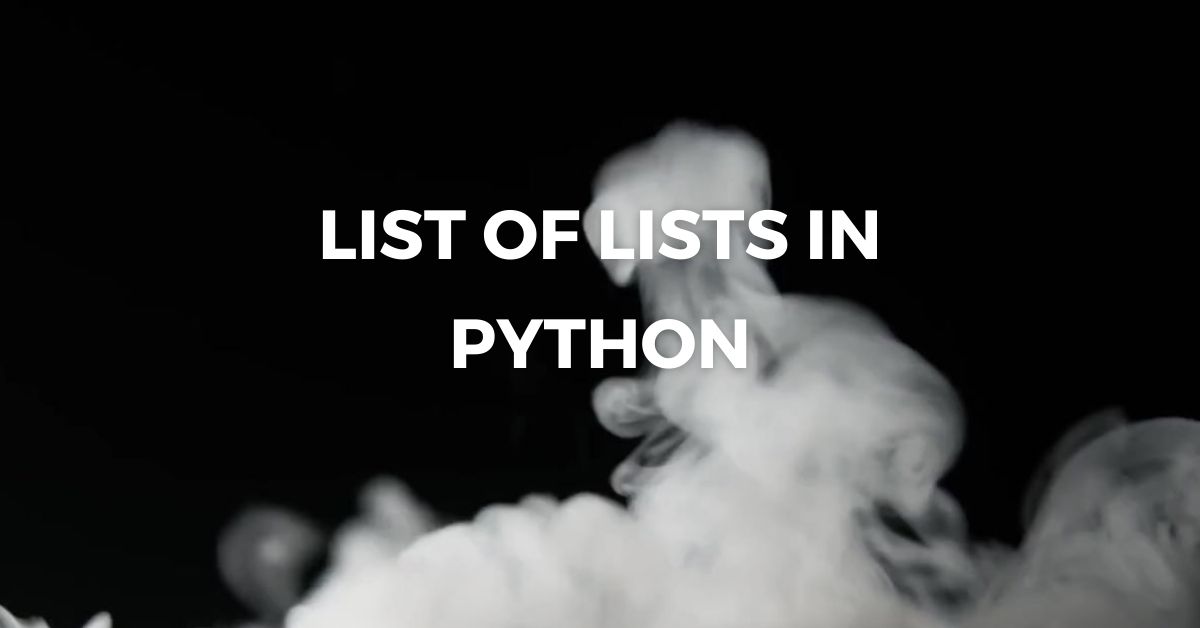
In Python, lists serve as containers for sequentially storing data elements. This discussion explores the creation and manipulation of a list of lists in Python, maintaining focus on operations such as sorting, traversing, and reversing to effectively manage and preserve the structure and content within a list of lists.
What Is a List of Lists in Python or list within a list python
Terms “list of lists” and “list within a list” in Python generally refer to the same concept. A list of lists in Python refers to a data structure where a list contains other lists as its elements. It facilitates the storage of multidimensional data, making it a crucial concept in Python programming.
Create a List of Lists in Python
Creating a list of lists involves placing several lists within another list. It is the simplest way to form a nested list structure.
list_of_lists = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
Each inner list can have different sizes, facilitating the creation of non-uniform data structures.
List of Lists Using the append() Method in Python
The append()
method helps in adding a new list to an existing list of lists.
list_of_lists.append([10, 11, 12])
Here, we added a new list to the end of list_of_lists
.
Using List Comprehension in Python
List comprehension provides a concise way to create lists of lists, allowing you to create complex lists with a single line of code.
list_of_lists = [[i for i in range(j, j+3)] for j in range(0, 9, 3)]
In this example, we created a list of lists using a nested loop within the list comprehension.
Access Elements in a List of Lists in Python
Accessing elements in a list of lists requires specifying the indices of both the outer and the inner list.
print(list_of_lists[0][1]) # Outputs: 2
Here, we accessed the second element (index 1) of the first list (index 0).
Traverse
Traversing a list of lists is generally done using nested loops, which allow you to iterate over each element in every list contained within the outer list.
for lst in list_of_lists:
for elem in lst:
print(elem, end=" ")
print()
In this script, we used two nested loops to iterate over each element in every inner list.
Delete an Element
To delete an element from a specific list within a list of lists, you can use the del
statement, mentioning the index of the element to be deleted.
del list_of_lists[0][1] # Deletes the element 2
Here, we deleted the second element of the first list.
Delete an Element Using the pop() Method
The pop()
method removes an element from a specified index and returns it, allowing you to both remove and get the element at the same time.
list_of_lists[0].pop(1) # Removes and gets the element 2
In this line, we used pop()
to remove and retrieve the second element from the first list.
Remove an Element Using the remove() Method
Using the remove()
method, you can delete the first occurrence of a specified value from a list.
list_of_lists[0].remove(3) # Removes the element 3
This script removes the element with the value 3 from the first list.
Flatten List of Lists in Python
Flattening refers to converting a list of lists into a single list that contains all the elements of all the inner lists.
flattened_list = [item for sublist in list_of_lists for item in sublist]
This list comprehension iterates over each element in each inner list, creating a new list that contains all these elements.
Reverse List of Lists in Python
Reversing a list of lists means reversing the order of the inner lists but not the individual elements within those lists.
list_of_lists.reverse()
After executing this code, the last list in list_of_lists
will become the first, and so on.
Reverse the Order of Inner List in List of Lists in Python
To reverse the order of the elements within each inner list, you can use a loop to reverse each list one by one.
for lst in list_of_lists:
lst.reverse()
In this script, we reversed the order of elements in every inner list.
Sort
Sorting a list of lists can be done based on various criteria such as the first element of each list, the sum of elements in each list, etc.
list_of_lists.sort(key=lambda x: x[0])
Here, we sorted the list of lists based on the first element of each inner list.
Sort Using the sort() Method
Using the sort()
method, you can sort the elements of each inner list individually.
for lst in list_of_lists:
lst.sort()
In this example, we iterated over each list and sorted them individually.
Sorting Using the sorted() Function
The sorted()
function can be used to obtain a new list that is sorted, without modifying the original list.
sorted_list_of_lists = [sorted(lst) for lst in list_of_lists]
Here, we created a new list of lists with each inner list sorted.
Concatenate
To concatenate two lists of lists, you can use the +
operator to merge them into a single list of lists.
list_of_lists1 = [[1, 2], [3, 4]]
list_of_lists2 = [[5, 6], [7, 8]]
concatenated_list_of_lists = list_of_lists1 + list_of_lists2
In this script, we merged two lists of lists into one.
Copy
Copying a list of lists can be achieved using the copy()
method, but it only creates a shallow copy.
copied_list_of_lists = list_of_lists.copy()
This means that the inner lists are not copied, but are referenced.
Shallow Copy
A shallow copy can also be created using the list()
constructor, which only creates a new outer list, keeping references to the inner lists.
shallow_copy = list(list_of_lists)
Modifying the inner lists in the shallow copy will affect the original list.
Deep Copy
A deep copy creates a new list of lists with new inner lists, not just references. You can create a deep copy using the deepcopy()
function from the copy
module.
from copy import deepcopy
deep_copy = deepcopy(list_of_lists)
With a deep copy, changes to the inner lists won’t affect the original list of lists.
Conclusion
Working with lists of lists in Python opens up numerous opportunities for handling complex data structures. In this guide, we explored how to create, access, modify, and manipulate lists of lists using various methods and functions. Equipped with this knowledge, you can now effectively work with multidimensional data in Python.
Read More:
1: Python Parallel For Loop
2: Python Hangman Code
3: Python List of Dictionaries
- How to Fix – TypeError: only size-1 arrays can be converted to Python scalars - 16 October 2023
- How to Implement d’wave qbsolv in Python - 16 October 2023
- Resolve Javascript error: ipython is not defined - 15 October 2023