Python Parallel For Loop
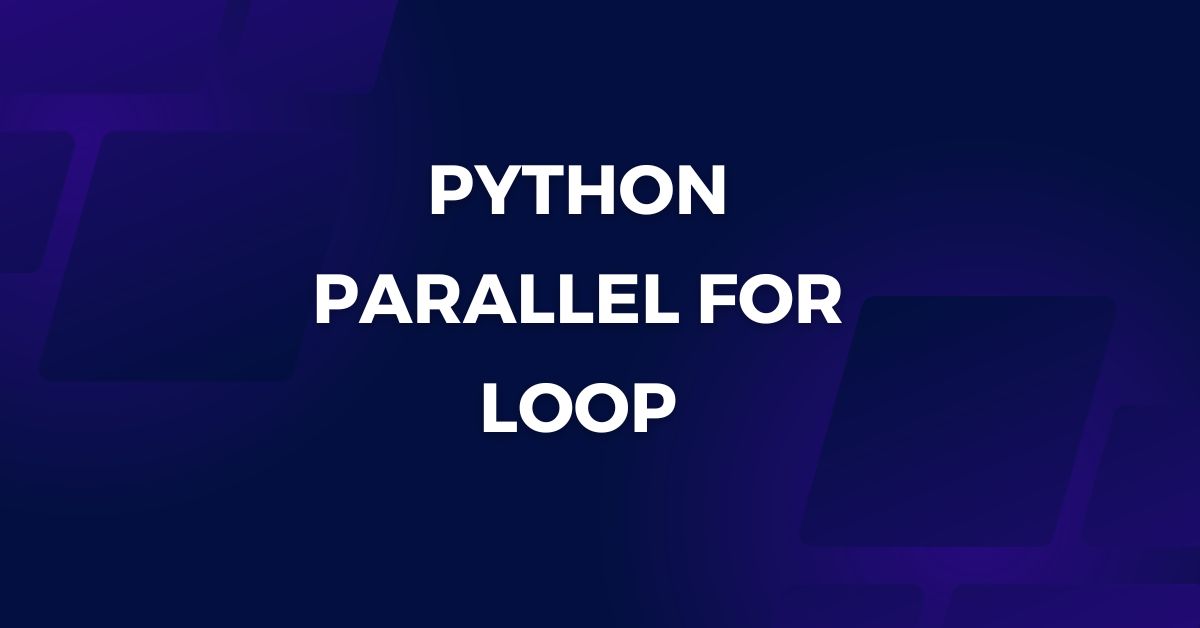
In Python, the for-loop is a powerful tool utilized to iterate over elements, such as items in a list or characters in a string. However, when working with a substantial amount of data, these iterations can become a bottleneck, significantly slowing down your code execution. This is where the Python parallel for-loop comes into the play, utilizing parallel processing to facilitate the concurrent execution of these iterations, helping you to run your code faster and more efficiently.
In the coming sections, we’ll cover the parallel execution of for-loops using Python’s multiprocessing module, helping you transform your serial loop into a Python parallel for-loop powerhouse.
Prerequisite (Prepare Your Code Before We Make it Parallel)
Before diving into the world of parallel computing, it is vital to dissect your existing code and identify which segments are independent and can be executed concurrently. Parallel execution shines when applied to tasks that are CPU-intensive and non-dependent on each other.
Get ready by installing Python and setting up a proper coding environment that supports multiprocessing.
How To Make For-Loop Parallel With Pool
To initiate parallel execution in Python, we will leverage the multiprocessing.Pool
class, which allows us to manage a pool of worker processes. Let’s start by importing the necessary module:
from multiprocessing import Pool
Example of Parallel For-Loop with map()
Serial For-Loop with map()
Before diving into parallel for-loops, let’s understand how a serial for-loop works with the map
function.
def square(n):
return n * n
numbers = range(10)
result = map(square, numbers)
print(list(result))
In this script, the square
function is applied to each element in the numbers range sequentially. The outcome will be a list of squared values, achieved through individual and independent operations on each element.
Parallel For-Loop with map()
To harness the power of parallel processing, we can modify the above script slightly by introducing Pool().map()
:
with Pool() as pool:
result = pool.map(square, numbers)
print(list(result))
Here, the iterable (numbers range) is divided into several chunks, with each chunk assigned to a separate process in the pool, speeding up the computational task substantially by running them in parallel.
Parallel For-Loop with map() and No Return Values
Not all functions return values. Sometimes, you might have a function that writes data to a file or sends information over a network. Even in these cases, using parallel for-loops can be beneficial as it can speed up the overall process.
Example of Parallel For-Loop with starmap()
When your function requires multiple parameters, starmap()
comes into play. It essentially allows you to pass an iterable of argument tuples to the function.
def power(base, exponent):
return base ** exponent
arguments = [(2, 2), (2, 3), (2, 4)]
with Pool() as pool:
result = pool.starmap(power, arguments)
print(result)
In this script, each tuple in the “arguments” list is unpacked to supply the parameters to the power
function, which is then executed in parallel, showcasing the true versatility of parallel for-loops in handling multi-parameter functions.
Example of Parallel For-Loop with imap()
Sometimes, we may prefer to get the results as soon as they are ready. The imap()
method comes handy in such scenarios. It returns an iterator that yields results in a manner that respects the order of the input iterable.
with Pool() as pool:
result = pool.imap(square, numbers)
print(list(result))
Note that imap
maintains the order of the results, so the first output would correspond to the first input, and so on. This is particularly useful when the order of the results matters.
Advanced Scenarios and Examples
Now that we have seen basic examples, let’s dive deeper into some more advanced scenarios where parallel for-loops can be beneficial.
Example with File Operations
Consider a scenario where we have a list of files that we want to read and process in parallel to save time. Here is how we can do it:
def read_file(file_path):
with open(file_path, 'r') as file:
data = file.read()
# Perform some operations on data (omitted for simplicity)
return data
file_paths = ['path1.txt', 'path2.txt', 'path3.txt']
with Pool() as pool:
results = pool.map(read_file, file_paths)
In this script, each process reads a different file, allowing the IO-bound operations to run in parallel and save time.
Example with Network Operations
Similarly, if we have network operations that can run independently, we can parallelize them to speed up the total execution time. Here is an illustrative example where we fetch data from different URLs:
import requests
def fetch_url(url):
response = requests.get(url)
return response.content
urls = ['https://www.url1.com', 'https://www.url2.com', 'https://www.url3.com']
with Pool() as pool:
results = pool.map(fetch_url, urls)
In this example, each URL is fetched in parallel, potentially reducing the total fetching time.
Common Questions
How To Set The Number of Workers
Setting the number of worker processes in the pool is straightforward. Use the processes
argument while initializing the Pool
class:
with Pool(processes=4) as pool:
# Your code here
How Many Workers Should I Use?
Identifying the optimal number of worker processes is crucial. A good starting point is to use as many workers as there are CPU cores. However, for IO-bound tasks, even a higher number of workers might be beneficial.
Shouldn’t I Set the Number of Workers to Be 1 Less Than The Number of CPUs?
While it may seem logical to leave one core free, it is generally not necessary, as the Python multiprocessing
module is quite efficient in managing processes and utilizing CPU resources optimally.
What is a Logical vs Physical CPU?
Understanding the difference between a physical and a logical CPU is important. A physical CPU is a hardware unit in your computer, while a logical CPU represents the abilities of a physical CPU to perform parallel operations, thanks to technologies like hyper-threading.
How Many CPUs Do I Have?
To find out the number of CPUs, you can use the os.cpu_count()
method from the os
module:
import os
os.cpu_count()
This function returns the number of logical CPUs, giving you a hint on the maximum number of worker processes you can use effectively.
What If I Need
to Access a Shared Resource?
When multiple processes need to access a shared resource, it is important to synchronize the access to prevent data corruption or other issues. Python’s multiprocessing
module offers several synchronization primitives like Lock
, Event
, and Condition
to help you in achieving safe access to shared resources.
What Types of Tasks Can Be Made Parallel?
Tasks that are CPU-intensive and can be broken down into independent subtasks are great candidates for parallelization. However, IO-bound tasks, such as file reading/writing or network operations, can also benefit from parallel execution, especially when the operations are independent and can be performed concurrently.
Further Reading
To delve deeper into the world of Python parallel processing, consider the following resources:
- Python’s multiprocessing documentation: Python Multiprocessing
- Dr. Jeff Heaton’s comprehensive YouTube guide: Jeff Heaton’s YouTube Guide
Takeaways
Parallelizing for-loops in Python using the multiprocessing
module can be a game-changer, significantly reducing the execution time of your scripts. This guide has equipped you with the foundational knowledge and examples to start implementing parallel for-loops in your Python projects, paving the way for faster and more efficient code.
Remember to always verify the correctness of your parallel code and to handle shared resources with caution to prevent unwanted side effects. With these tips in mind, you are now ready to unlock the full potential of your CPU and take your Python coding skills to the next level.
Read More:
Python – Import from Parent Directory
Python was not found; run without arguments
- How to Fix – TypeError: only size-1 arrays can be converted to Python scalars - 16 October 2023
- How to Implement d’wave qbsolv in Python - 16 October 2023
- Resolve Javascript error: ipython is not defined - 15 October 2023