How do I print an empty line in Python?
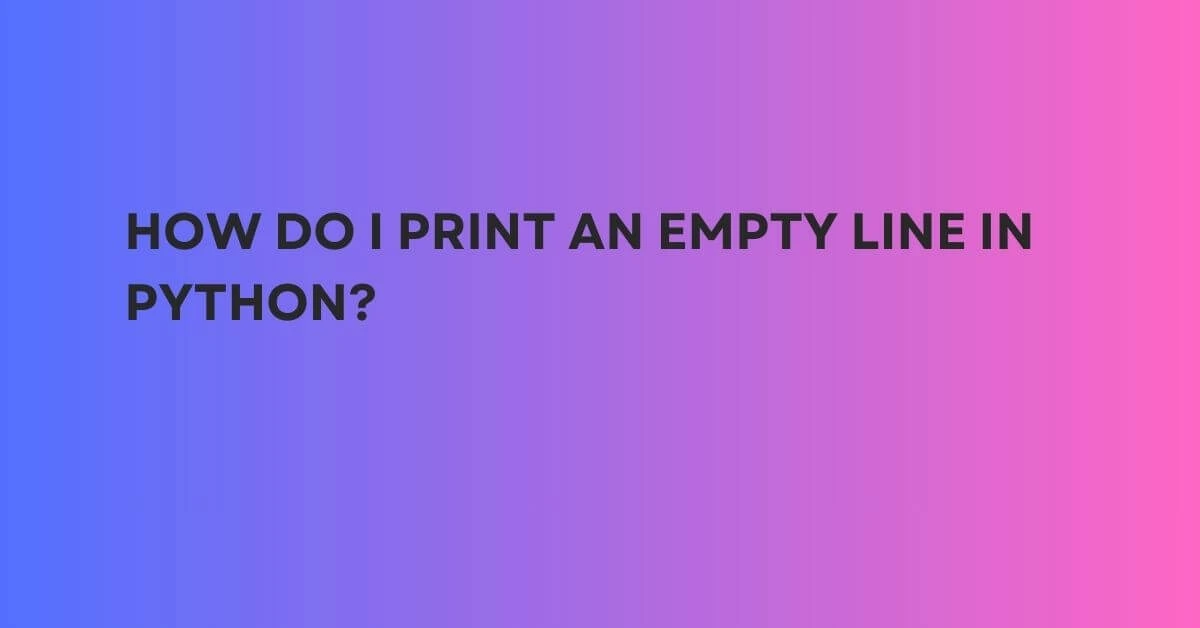
Printing an empty line or a blank space is a very common task in programming. If you want to separate the output of your program or add some whitespace for readability, printing an empty line is a simple way to achieve this. In this article, We will learn multiple ways to print an empty line in Python.
To print an empty line in Python is achieved by calling the print()
function with an empty string as its argument, like print("")
. This will print a new line character to the console, effectively creating an empty line. You can also use the escape character “\n"
to print a newline directly, like : print("\n")
.

Python supports various ways to print an empty line and we will discuss maximum all the simple ways to achieve this.
Methods to print an empty line
Using the print()
Function with No Arguments
If you think of an empty line in the output, the first thing that hit your mind is the print()
function. When you call print()
with no arguments, it prints a newline character, which moves the cursor to the next line.
Example
print("Learn & Share")
print()
print("Help to learn by sharing our mistakes")
This code will print a single empty line to the console.
Output:
Learn & Share Help to learn by sharing our mistakes
Using the print()
Function with an Empty String
Similar to the previous method, We can print an empty line in Python by using the print()
function with an empty string as its argument. An empty string is a string with no characters which does not print anything in the console. So, when we pass an empty string to the print()
function, it still prints a newline character, which moves the cursor to the next line.
Example:
print("Learn & Share")
print("")
print("Help to learn by sharing our mistakes")
This code will also print a single empty line to the console.
Output:
Learn & Share Help to learn by sharing our mistakes
Using the Escape Character \n
The escape character “\n"
represents a newline character in Python. This can be used along with the string that you are passing to the print()
function as below
Example:
print("Learn & Share \n")
print("Help to learn by sharing our mistakes")
This code will print a single empty line to the console after printing the string, just like in the previous examples.
Output:
Learn & Share Help to learn by sharing our mistakes
Using print()
with the end
Parameter
This is as same as the above print function Because the value of the end parameter is “\n
” by default, Which means, After the print statement, it will go to the next line, As same as the initial method. But it is good to know that you can able to change the default value as below
Example
print("Learn & Share")
print("", end="\n")
print("Help to learn by sharing our mistakes")
This code will print a single empty line to the console, just like in the previous examples.
Output:
Learn & Share Help to learn by sharing our mistakes
Using the sys
Module
The sys
the module provides access to system-specific parameters and functions. One of these functions is sys.stdout.write()
, This helps to write strings passed as input in the console, By using the escape character “\n"
along with the write function, We can create an empty line
import sys print("Learn & Share") sys.stdout.write("\n") print("Help to learn by sharing our mistakes")
This code will print a single empty line to the console, just like in the previous examples.
Output:
Learn & Share Help to learn by sharing our mistakes
Using the os
Module
The os
module provides a way to interact with the operating system. One of its functions is os.write()
, which writes a string to a file descriptor (a number that represents an open file or stream). The file descriptor for the console is 1, so you can use os.write(1, "\n")
to write a newline character to the console. Here’s an example:
import os
print("Learn & Share")os.write(1, b"\n")
print("Help to learn by sharing our mistakes")
This code will print a single empty line to the console, just like in the previous examples. Note that os.write()
requires a bytes object as its second argument, so we use b"\n"
instead of "\n"
.
Output:
Learn & Share Help to learn by sharing our mistakes
Using print()
with the sep
Parameter
The sep
the parameter is used along with the print()
function in Python that specifies the separator to use between multiple arguments passed to the print()
function. By default, the sep
the value is set to " "
, which means that a space character will be used to separate the arguments.
Example:
print("Learn", "Share", sep=" & ")
Above code will include the “&” in between the strings as below
Learn & Share
As you can see, we’ve used sep=" & "
to specify that a symbol ” & “should be used to separate the two string arguments.
Having said that, We can use this sep to include an empty line between strings. For that, you can just add “\n
” escape character in the sep parameter two times to go to the next line and create an empty line as below
Example:
print("Learn", "Share", "Knowledge", sep="\n\n")
This code will output the following string to the console:
Learn Share Knowledge
As you can see, the three string arguments are concatenated without any separator between them.
Print multiple empty lines in the Output
Additionally, If you want to print multiple empty lines in the output/console, you can try the below method.
Using Multiplication Operator
We can use the Multiplication Operator “*” to multiply the number of empty lines in the output like ('/n' * 10
)
Example:
print("Learn & Share" , '\n' * 4) print("Help to learn by sharing our mistakes")
The above code will print 4 empty lines between two strings, Below is the output of the code
Learn & Share Help to learn by sharing our mistakes
Conclusion
In Conclusion, There are multiple ways to add an empty line in the output. Out of which, the most commonly used method is to call the print()
function, either an empty print function or with an empty string (discussed above) which prints a newline in the output. We have also discussed many alternative approaches like escape character “\n"
with the print function or by using sys
or os
module to achieve an empty line. At last, we discussed the sep
parameter of the print()
function, which can be used to specify the separator between multiple arguments passed to print()
. All of these techniques are useful tools for controlling the output of your Python programs.
Read More:
How to convert RGB to Hex and Hex to RGB code in Python
How to Make Jarvis With Dialogflow and Python
How do I reinstall Anaconda Python
How to Nest a Dictionary in a List?
Coding Spell | Python Journey | About Us
- How To Find the Length of a List in Python - 16 October 2023
- Python Program to Swap Two Variables - 16 October 2023
- How to Check if a Variable is Null in Python - 14 October 2023